Exercise 4 - Award Grades
In Digital Solutions, the final award of letter grades based on marks changes slightly each year. For example, a student in 2020 with a final mark of 66 was awarded a 'B' grade; whereas a student with the same mark in 2021 was awarded a 'C' grade.
Mark ranges to letter grades also change between subjects. For example, a student in General Mathemetics in 2021 with a final mark of 80 was awarded a 'B' grade; whereas a student with the same mark in 2021 in English was awarded an 'A' grade.
With that said, for the following algorithm, work on these mark to grade thresholds:
Mark Range (inclusive) | Grade |
---|---|
44 or lower | D |
45 to 65 | C |
66 to 82 | B |
83 to 100 | A |
An algorithm is required to read in a list (a.k.a., 'array') of scores, and assign letter grades to these, based on the thresholds above. The end result should look like this:
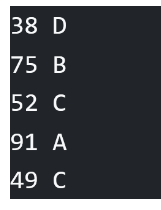
The algorithm has been started but the awardGrade
module needs finishing:
BEGIN awardGrade(score) grade = '' IF score <= 44 THEN ... ENDIF RETURN grade END BEGIN scores = [38, 75, 52, 91, 49] FOR counter = 0 TO LENGTH(scores) score = scores[counter] grade = awardGrade(score) OUTPUT score, grade NEXT counter ENDFOR END
Finish coding the awardGrade
module here in Python once you have finished planning it above:
def awardGrade(score): grade = '' if score <= 44: #keep typing here... return grade scores = [38, 75, 52, 91, 49] for score in scores: grade = awardGrade(score) print(score, grade)