Python
Python → download → How to install Python (optional: VS Code)
console applications | ||||
---|---|---|---|---|
# | console 1 | console 2 | console 3 | console adv |
input output | iteration while | string manipulation | object oriented programming theory | |
data types | iteration for | dictionaries | class, instance and method | |
random numbers | recreate challenge iii | sets | constructor and instance variable | |
branching if | recreate challenge iv | tuples | class variables | |
branching if else | iteration nested | exercises E | inheritance | |
recreate challenge i | exercises B | exercises F | polymorphic override and private | |
branching and or not | exercises C | functions | ||
recreate challenge ii | lists | recursion | ||
nested & branching elif | recreate challenge v | importing separate files | ||
exercises A | exercises D | files R/W |
roulette
card draw |
|
mc test i answer sheet |
mc test ii answer sheet |
exceptions |
unsorted | |||
---|---|---|---|
# | object-oriented | web scraping | other |
card deck | installation | trader game | |
doc types | first scrape | access | |
variable scope | bs4 functions | unsorted | |
multiple classes | combining data sources | unsorted | |
autoteller console | legality of scraping |
spiral hello |
|
merge (recursive) sort | HTML pages for web scraping |
Careflight data Glass artworks data |
more practise | |||
---|---|---|---|
# | challenge or activity | ||
exam 1 revision sheet | |||
exam 2 revision sheet | |||
exam 3 revision sheet | |||
Coins Challenge | |||
Dice Challenge | |||
Multicopier Context | |||
Bank Context | |||
Fast Food Context |
print("Caesar Cipher") plain_text = input("Enter plaintext message to encrypt: ") cipher_text = [] for character in list(plain_text): #normalise characters for shifting by using a base 26 (0-25) ordinal system: plain_ordinal = ord(character) - ord("A") if character.isupper() else ord(character) - ord("a") #shift +3, and wrap using Modulo operator (%) if over length of alphabet (26): cipher_ordinal = (plain_ordinal + 3) % 26 #return cipher as all uppercase to avoid patterns, as randomness makes for stronger encryption: cipher_text.append( chr( cipher_ordinal + ord("A") ) ) print("Encrypted ciphertext:", "".join(cipher_text))
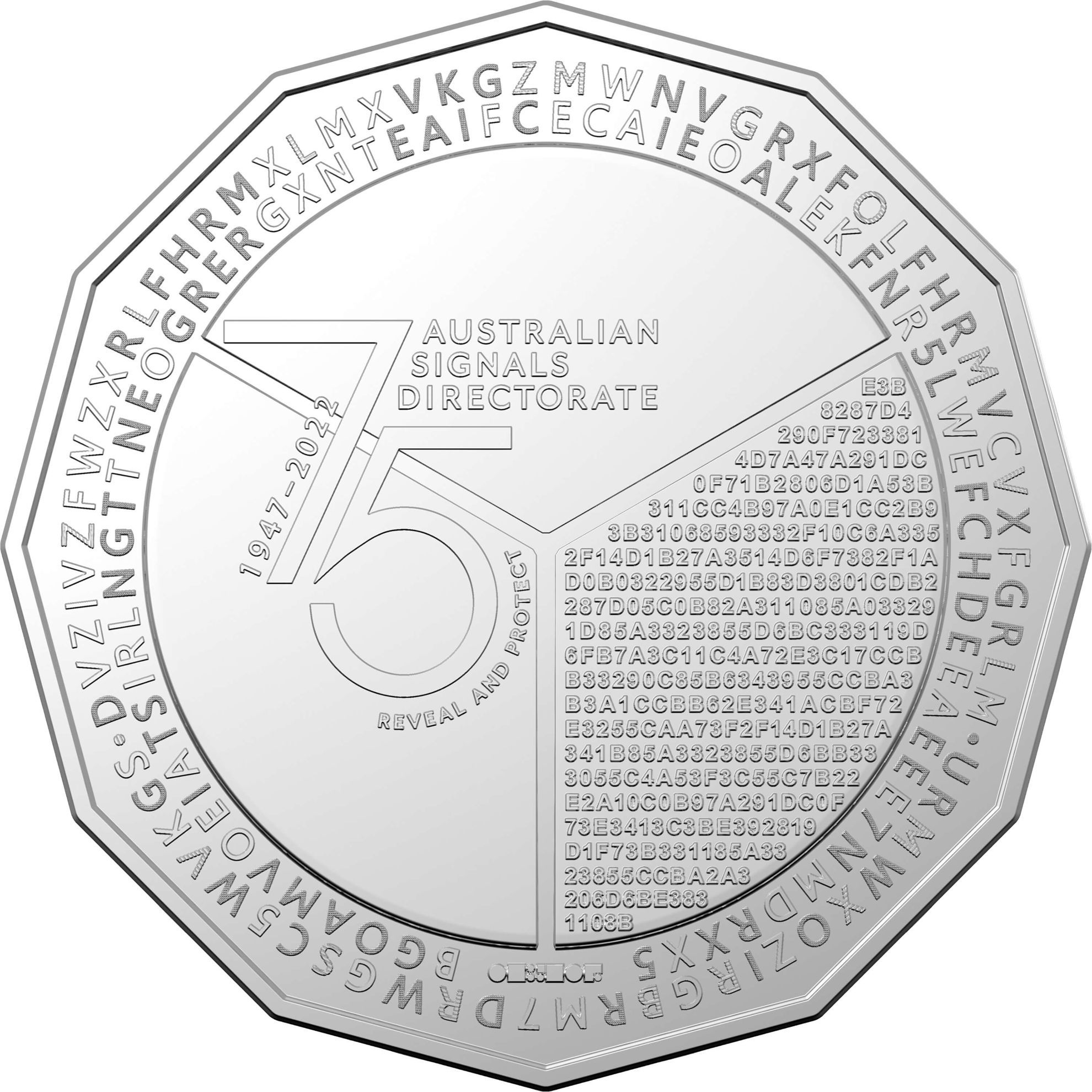
key = "A5D75" #Australian Signals Directorate 75th Anniversary fifty_cent_coin_hex_chars = """ E3B 8287D4 290F723381 4D7A47A291DC 0F71B2806D1A53B 311CC4B97A0E1CC2B9 3B31068593332F10C6A335 2F14D1B27A3514D6F7382F1A D0B0322955D1B83D3801CDB2 287D05C0B82A311085A03329 1D85A3323855D6BC333119D 6FB7A3C11C4A72E3C17CCB B33290C85B6343955CCBA3 B3A1CCBB62E341ACBF72 E3255CAA73F2F14D1B27A 341B85A3323855D6BB33 3055C4A53F3C55C7B22 E2A10C0B97A291DC0F 73E3413C3BE392819 D1F73B331185A33 23855CCBA2A3 206D6BE383 1108B""".replace('\n', '').replace('\r', '') #remove new line chars def extend(key, length): #to extend key to length of encrypted text return (key * (length//len(key) + 1))[:length] def decrypt(a, b): #to XOR two hex (base 16) strings of the same length return "".join(["%x" % (int(x,16) ^ int(y,16)) for (x, y) in zip(a, b)]) keyblock = extend(key, len(fifty_cent_coin_hex_chars)) result = decrypt(fifty_cent_coin_hex_chars, keyblock) plaintext = bytes.fromhex(result).decode("ASCII") print(plaintext)