Hello World
simplest.py
import PySimpleGUI rows = [ [PySimpleGUI.Text("Welcome")], [PySimpleGUI.ReadFormButton("Click me")] ] form = PySimpleGUI.FlexForm("This is a form.") form.Layout(rows) button, value = form.Read()
simpler.py
import PySimpleGUI as PSG rows = [ [PSG.Text("Welcome")], [PSG.ReadFormButton("Click me")] ] form = PSG.FlexForm("This is a form.") form.Layout(rows) button, value = form.Read()
simple.py
import PySimpleGUI as PSG rows = [ [PSG.Text("Welcome")], [PSG.ReadFormButton("Click me"), PSG.Cancel("Exit")] ] form = PSG.FlexForm("This is a form.") form.Layout(rows) button, value = form.Read()
helloWorld.py
import PySimpleGUI as PSG message = PSG.Text("Welcome") rows = [ [message], [PSG.ReadFormButton("Click me")] ] form = PSG.FlexForm("This is a form.") form.Layout(rows) button, value = form.Read() if button == "Click me": message.Update("Hello World")
helloWorldRefined.py
import PySimpleGUI as PSG message = PSG.Text("Welcome") rows = [ [message], [PSG.ReadFormButton("Click me")], [PSG.ReadFormButton("Clear"), PSG.Cancel()] ] form = PSG.FlexForm("This is a form.") form.Layout(rows) while True: button, value = form.Read() if button == "Click me": message.Update("Hello World") elif button == "Clear": message.Update("") else: break
- Create a GUI application that says "What do you watch on TV?" when it loads. Make it have a button that says "Netflix", and when I push the Netflix button, the "What do you watch on TV?" text is replaced with the word
Netflix
. - Add a "Stan" and "Twitch" button to the app you just made, which do the same thing as the "Netflix" button (except these replace the "What do you watch on TV?" text with
Stan
andTwitch
respectively). - Add an "Exit" button, a "Clear" button and a "Reset" button to the program you just made. Put all the TV buttons on one row, and the "Exit", "Clear" and "Reset" buttons on a different row underneath. Make the "Exit" button
break
out of the main loop when i click it. Make the "Clear" button clear all text that is showing in the "What do you watch on TV?" text element. Make the "Reset" button show the original text when the app loads ("What do you watch on TV?"). An image of the final product is shown here:
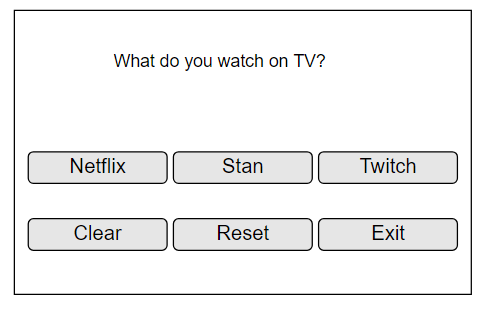