My First Button
myfirstbutton1.py
from PyQt6.QtWidgets import QApplication, QWidget, QPushButton app = QApplication([]) window = QWidget() myFirstButton = QPushButton("Gangster", window) myFirstButton.move(25, 25) window.show() app.exec() #main loop
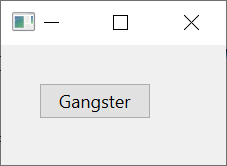
myfirstbutton2.py
from PyQt6.QtWidgets import QApplication, QWidget, QPushButton app = QApplication([]) window = QWidget() myFirstButton = QPushButton("Gangster", window) myFirstButton.move(25, 25) window.show() def doSomething(): #this receives SIGNAL, known as a SLOT print("You clicked gangster!") #send a 'signal' to a 'slot' using .connect: myFirstButton.clicked.connect(doSomething) #this sends SIGNAL app.exec() #main loop
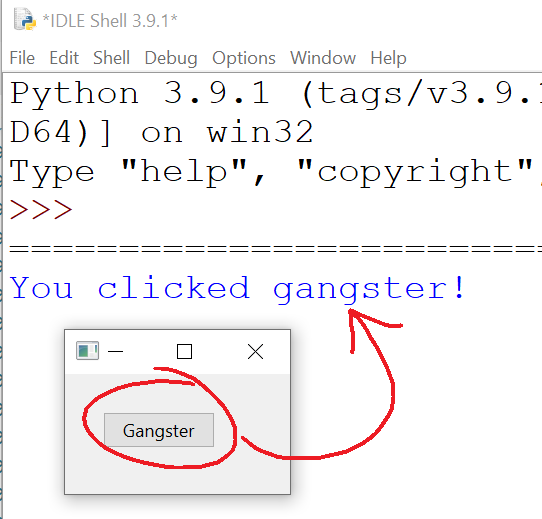
myfirstbutton3.py
from PyQt6.QtWidgets import ( QApplication, QWidget, QPushButton, QLabel ) app = QApplication([]) window = QWidget() myFirstButton = QPushButton("Gangster", window) myFirstButton.move(25, 25) myFirstLabel = QLabel("?????", window) myFirstLabel.move(25, 75) #50 pixels below the button myFirstLabel.setFixedWidth(200) myFirstLabel.setStyleSheet(""" background-color: yellow; font-size:24px; """) window.show() def doSomething(): myFirstLabel.setText("Stonks Mate!") myFirstButton.clicked.connect(doSomething) app.exec() #main loop
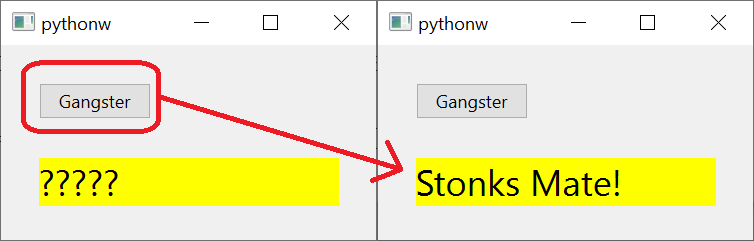
myfirstbutton4.py
from PyQt6.QtWidgets import QApplication, QWidget, QPushButton app = QApplication([]) window = QWidget() lightSwitch = QPushButton("Light Switch", window) lightSwitch.setCheckable(True) ### <-- LIGHT SWITCH STYLE BUTTON window.show() def switch(checked): text = "light switch state: " + str(checked) print( text ) lightSwitch.clicked.connect(switch) app.exec() #main loop
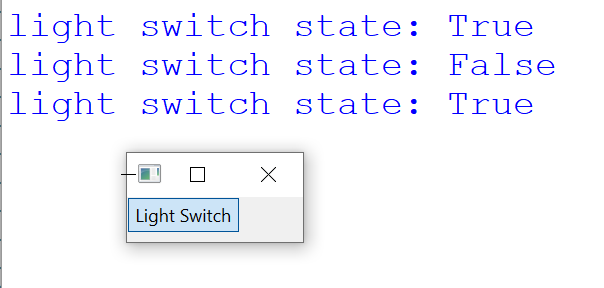