♦♣♥♠ Modified Yahtzee Challenge ♦♣♥♠
For this challenge we are going to make a modified version of the game Yahtzee:
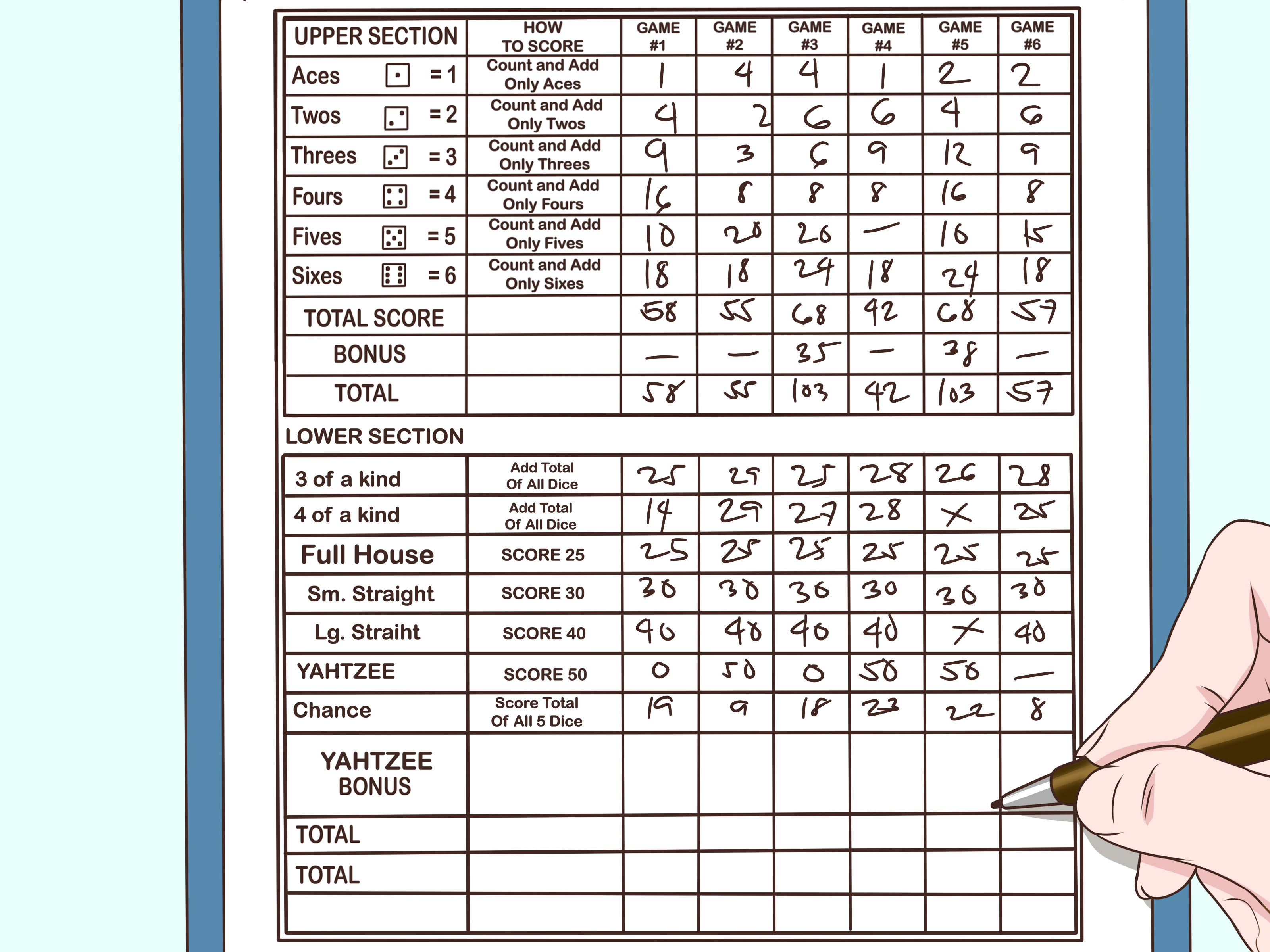
To complete this challenge, we will use a list to manage our dice rolls. Some code to help you get started with this is shown here:
import random #use a list to manage our 5 dice: five_dice = [] #roll 5 dice, one at a time: for roll in range(5): #each roll is between 1 and 6: dice_roll = random.randint(1,6) #join the roll to the list of dice: five_dice.append(dice_roll) #show the list of five dice: print(five_dice)
When you run this code, it will display the list of 5 dice as shown:
[3, 6, 3, 1, 4]
Your task is to process the list and determine if the following combinations exist. If more than one combination exists, the combination with the highest importance takes precedence. For example:
Combination | Example | Importance |
---|---|---|
No Pair | [2, 6, 4, 1, 3] |
1 |
One Pair | [2, 6, 2, 1, 3] |
2 |
Two Pair | [4, 1, 4, 5, 5] |
3 |
Three of a Kind | [4, 1, 4, 4, 6] |
4 |
Four of a Kind | [2, 2, 2, 3, 2] |
5 |
Full House | [5, 5, 2, 5, 2] |
6 |
Bottom Straight | [4, 3, 2, 5, 1] |
7 |
Top Straight | [5, 6, 3, 4, 2] |
8 |
Yahtzee | [4, 4, 4, 4, 4] |
9 |
Some examples of your code running are shown here. Example 1:
[2, 1, 4, 6, 5] No Pair
Example 2:
[6, 6, 2, 3, 6] Three of a Kind
♦♣♥♠ Extension Yahtzee Challenge ♦♣♥♠
Using your code above, simulate
n
number of rolls, where n
is an integer inputted by the user, and tally the results as follows:Number of rolls: 5 [4, 5, 1, 2, 2] [2, 6, 6, 6, 3] [5, 1, 5, 1, 3] [3, 2, 3, 6, 6] [4, 2, 1, 5, 3] No Pair: 0 One Pair: 1 Two Pair: 2 Three of a Kind: 1 Four of a Kind: 0 Full House: 0 Bottom Straight: 1 Top Straight: 0 Yahtzee: 0