Exercise 6 - Watch List
You are an investor looking to purchase cheap, energy efficient cryptocurrency. You have been given a list of cryptocurrencies and their exchange rates (in the variable exchangeRates), which you must parse to determine which currency to purchase.
The two rules that both must be satisfied before purchasing are:
- cryptocurrency must be exchanging below $1.50AUD
- cryptocurrency must be on the watch list for energy efficient crypto currency
The data for this task has been provided for you below (note - it has been redacted to make completing this task more manageable). The output should look something like this:
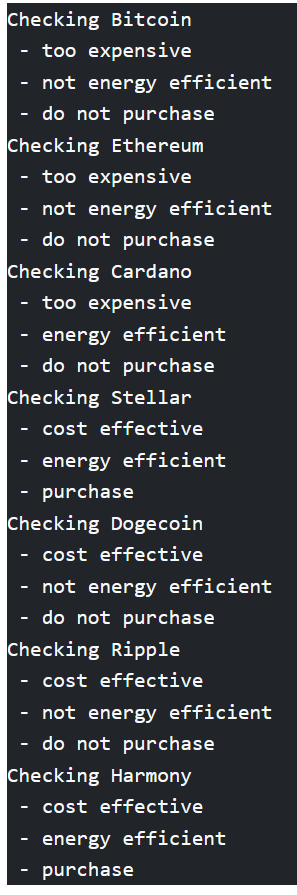
The algorithm must parse all of the cryptocurrencies in the exchangeRates variable, and make use of at least two sub-routines (measureCost
and checkEnergyEfficiency
). It has been started, but needs finishing:
energyEfficientCryptos = [ "SolarCoin", "Chia", "Cardano", "Stellar", "Harmony" ] exchangeRates = [ ("Bitcoin", 65460.31), ("Ethereum", 5272.41), ("Cardano", 1.87), ("Stellar", 0.38), ("Dogecoin", 0.24), ("Ripple", 1.19), ("Harmony", 0.34) ] BEGIN checkEnergyEfficiency(cryptocurrency) ... END BEGIN measureCost(cryptocurrency, exchangeRate) ... END BEGIN ... END
Code the measureCost
and checkEnergyEfficiency
modules you have planned above in Python:
energyEfficientCryptos = [ "SolarCoin", "Chia", "Cardano", "Stellar", "Harmony" ] exchangeRates = [ ("Bitcoin", 65460.31), ("Ethereum", 5272.41), ("Cardano", 1.87), ("Stellar", 0.38), ("Dogecoin", 0.24), ("Ripple", 1.19), ("Harmony", 0.34) ] def checkEnergyEfficiency(cryptocurrency): #checkEnergyEfficiency() module code here def measureCost(cryptocurrency, exchangeRate): #measureCost() module code here #MAIN ALGORITHM: for (crypto, cost) in exchangeRates: print("Checking", crypto) costEffective = measureCost(crypto, cost) energyEfficient = checkEnergyEfficiency(crypto) if costEffective and energyEfficient: #...