bubble sort
sorting a list of numbers into order...
unoptimised algorithm:
start from the beginning of the list compare every adjacent pair swap their position if they are not in the right order repeat these steps until the list is sorted
and in java:
public class bubbleSort{ public static void main(String[] args) { int[] array = new int[]{35, 71, 1, 10, 4}; boolean swapsies = true; int temp; while(swapsies) { swapsies = false; for(int index=0; index<4; index++) { if(array[index] > array[index+1]) { swapsies = true; temp = array[index]; array[index] = array[index+1]; array[index+1] = temp; } } } for(int index=0; index<5; index++) { System.out.println(array[index]); } } }this is unoptimised, because the biggest numbers will "bubble" to the end each pass (look at the dark rectangles in the following gif)... so we dont pass through the whole array right to the end each time:
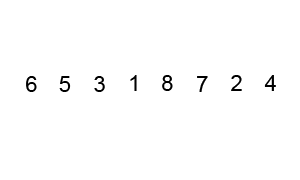
more optimised algorithm:
start from the beginning of the list compare every adjacent pair swap their position if they are not in the right order *after each iteration, compare one less element (the last one)* repeat these steps until the list is sorted
and in java:
public class bubbleSort{ public static void main(String[] args) { int[] array = new int[]{35, 71, 1, 10, 4}; boolean swapsies = true; int temp; while(swapsies) { //optimising bubble sort: int lastIndex = (array.length-1); //*********************** swapsies = false; for(int index=0; index<lastIndex; index++) { if(array[index] > array[index+1]) { swapsies = true; temp = array[index]; array[index] = array[index+1]; array[index+1] = temp; } } lastIndex--; } for(int index=0; index<5; index++) { System.out.println(array[index]); } } }