JavaScript Challenges #1 easy
Question 1: Create a JavaScript / HTML file that when i click a button, it changes the innerHTML
of a <h1>
tag, as shown in the following image:
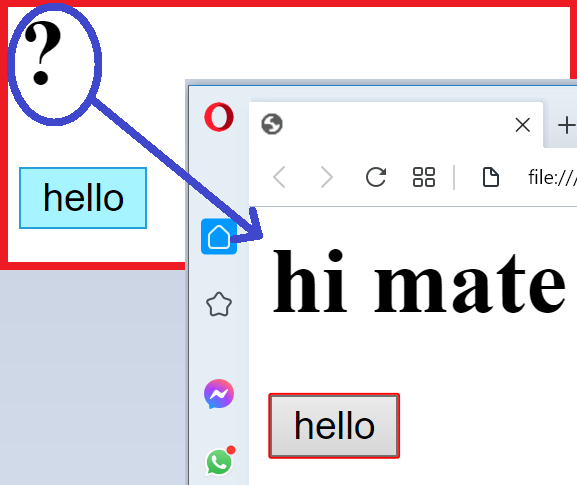
Question 2: Create the following file in VS Code, save it, and launch it in your favourite browser:
image changer.html
<img id="display" src="https://digisoln.com/bin.png"> <button onclick="change()"> change pic </button> <script> function change(){ printer = "https://digisoln.com/print.png"; document.getElementById("display").src = printer; } </script>
Question 3: With the HTML file you just created in Question 2, make the following changes:
- Change the image of the bin to a link to a dice showing the value of 1: https://digisoln.com/resources/dice/dice1.bmp
- Change the image of the printer to a link to a dice showing the value of 2: https://digisoln.com/resources/dice/dice2.bmp
- Test the page to make sure the image still changes after making your changes
Question 4: Create the following file in VS Code, save it, and launch it in your favourite browser:
Coin toss.html
<img id="coin" src="https://digisoln.com/resources/coins/heads.gif"> <button onclick="flip()"> flip coin </button> <script> var heads = "https://digisoln.com/resources/coins/heads.gif"; var tails = "https://digisoln.com/resources/coins/tails.gif"; function flip(){ number = Math.floor((Math.random() * 2) + 1) if (number == 1){ document.getElementById("coin").src = heads; } if (number == 2){ document.getElementById("coin").src = tails; } } </script>
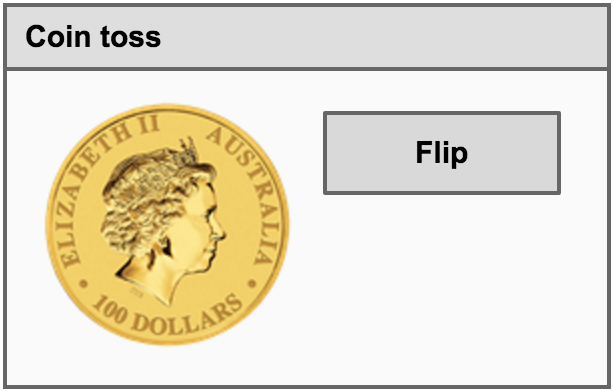
Question 5: The game you just made is Coin toss, and tosses a coin to reveal heads or tails (pictured above). Can you enhance this game by counting and displaying the number of heads and tails i flip? An image of the requirements and some starter code is shown below:
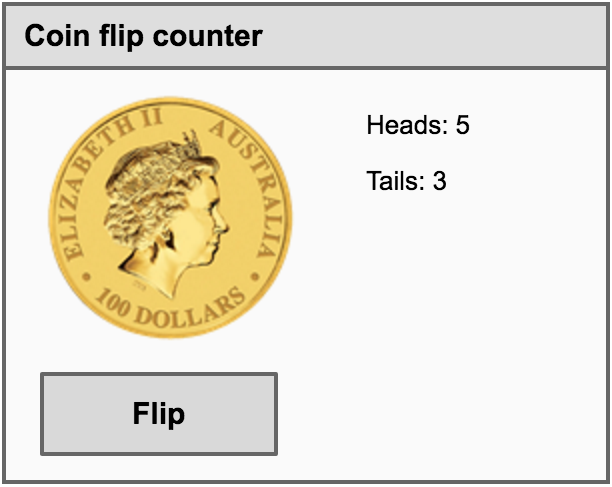
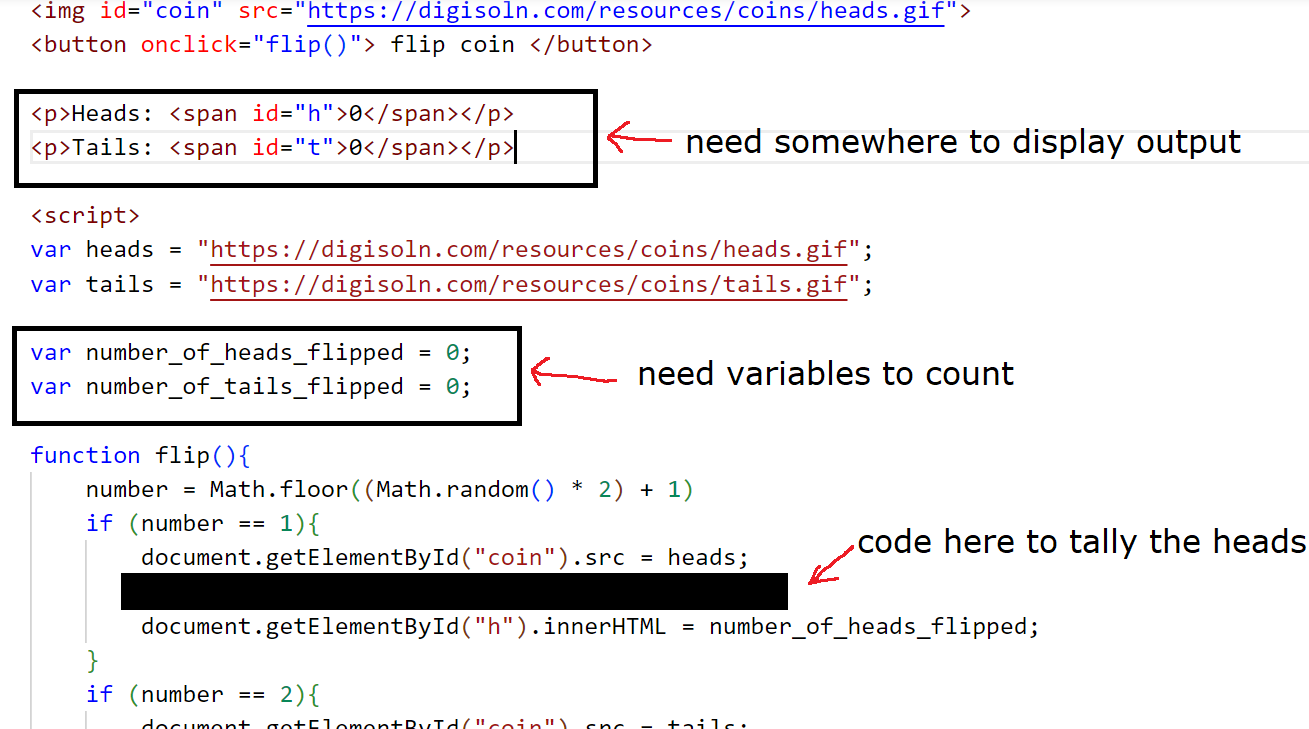
Question 6: Using the image links to dice from Question #3, and the random number code from Question #4, can you make a single dice roller HTML / JS file, as shown below:
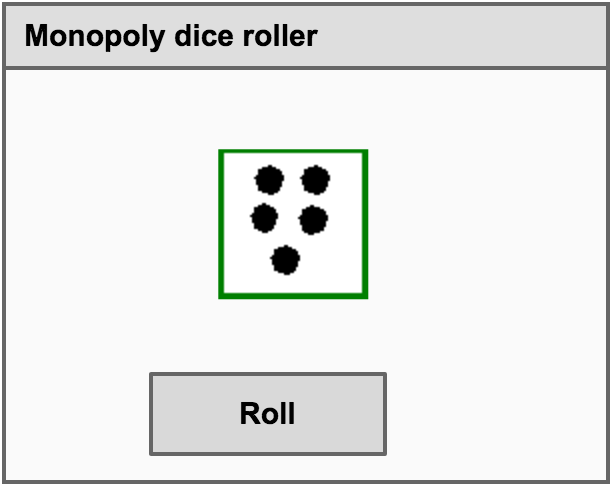
Hint: you may need to modify the random code to allow for 6 outcomes, e.g.: number = Math.floor((Math.random() * 6) + 1)