JavaScript Challenges #3 hard
Question 1: Recreate the card draw game using JavaScript within a HTML file, and launch it in your favourite browser. Each time i hit draw button, a new random card should appear:
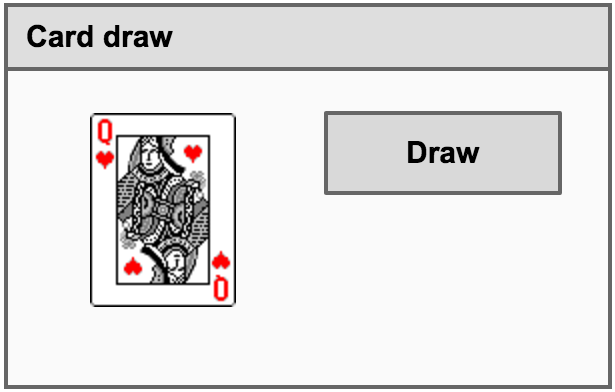
Hint: You can find online links to a full deck of cards here: https://digisoln.com/resources.html. There is also a JS file with some advanced card functions here which you are welcome to use in your assignments / games / challenge tasks: https://digisoln.com/resources/cards.js. An example of how to incorporate this external JS file can be found here:
<img id="image" src="https://digisoln.com/resources/cards/cardback.gif"> <style> table, tr, td { border: 1px black solid; } table { border-collapse: collapse; } </style> <table> <tr> <td id='name'></td> </tr> <tr> <td id='weight'></td> </tr> <tr> <td id='value'></td> </tr> <tr> <td id='suit'></td> </tr> </table> <script src="https://digisoln.com/resources/cards.js"></script> <script> reset(); // arranges a 52 deck of cards in order in memory shuffle(); // shuffles the deck of 52 cards var card1 = deal_card(); // takes next card from top of the deck document.getElementById("image").src = card1.url document.getElementById("name").innerHTML = card1.name document.getElementById("weight").innerHTML = card1.weight document.getElementById("value").innerHTML = card1.value document.getElementById("suit").innerHTML = card1.suit </script>
Question 2: Add four more cards to the previous program you just made in Question #1 to make a complete poker hand.
Hint: This challenge is Version 1 of the Card Draw Challenge. If you are enjoying working with playing cards, then you are welcome to pursue the Card Draw Challenges as a bonus activity:
Card Draw ChallengeQuestion 3: Re-create the following Maths Blaster game using parseInt
to get integer answers through the input text field. each time i hit New sum button it should generate a simple addition problem with two random numbers between 1 and 10:
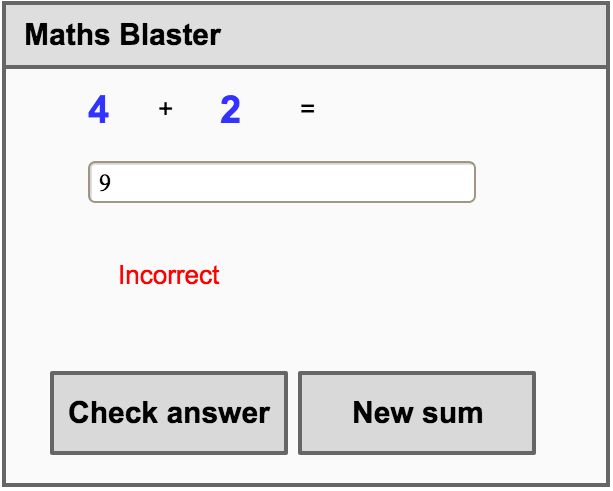
Question 4: For your game in Question #3, also include stat tracking for right and wrong answers as shown here:
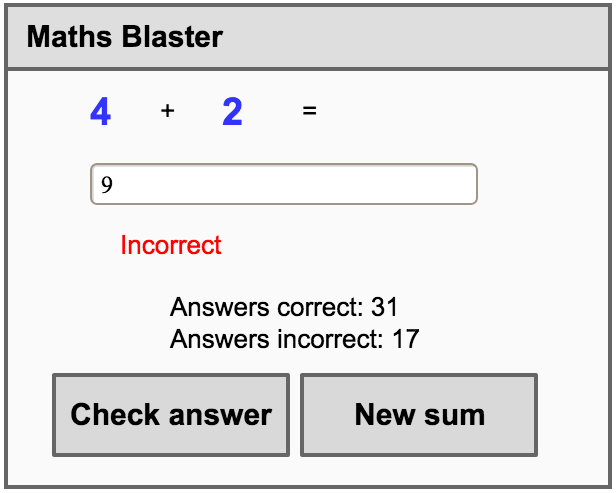
Question 5: For your game in Question #4, also include the ability to choose different question types (i.e., minus / divide / times), as shown here:
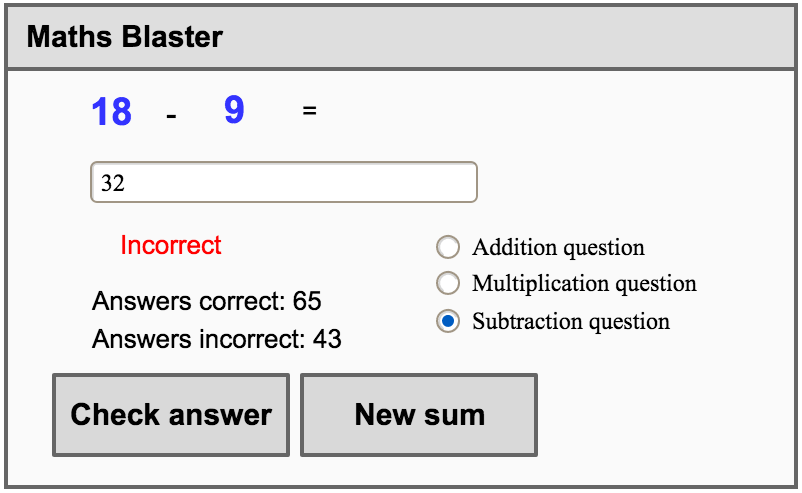
Question 6: In this link: Guess Next, there is a VC ("virtual credits") system implemented, where users can predict the outcome of these games and win credits. Each games costs VC / credits to play.
Guess Next games (with VC)Your challenge for this question is to implement a virtual credit system into either your cards, dice, coins, or maths game above, so that users can submit some VC in order to (potentially) win some back on a correct guess / answer.