JavaScript Challenges #2 medium
Question 1: Create the following game using JavaScript within a HTML file, and launch it in your favourite browser:
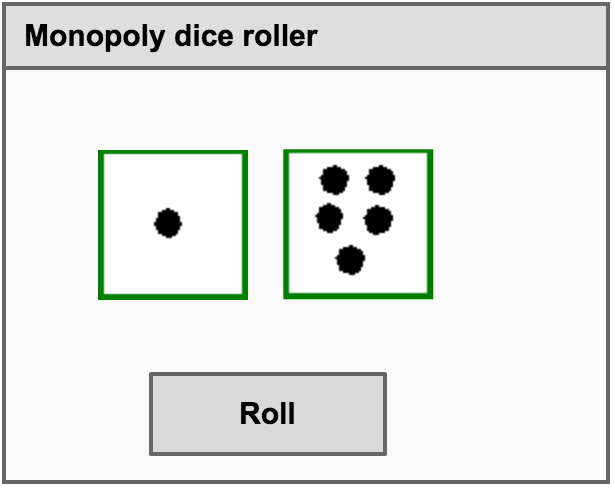
Question 2: Modify the code you wrote in Question #1 so that the sum of the two dice, as well as whether or not it is doubles, is displayed, as shown here:
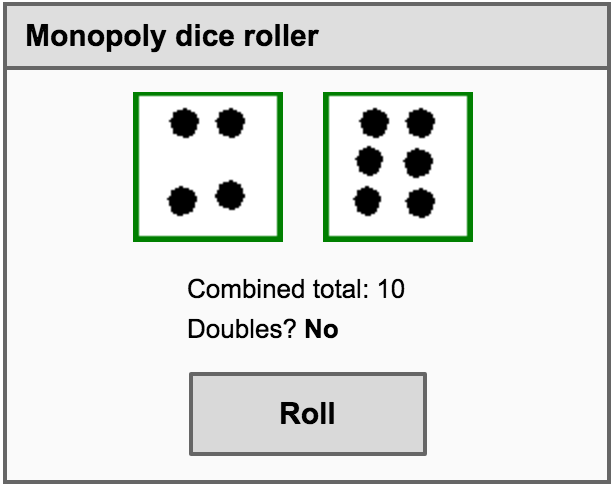
Question 3: Create the following file in VS Code, save it, and launch it in your favourite browser:
Guess which dice is going to be higher.html
<img id="dice1" src="https://digisoln.com/resources/dice/unknown.bmp"> <img id="dice2" src="https://digisoln.com/resources/dice/unknown.bmp"> <p>Which dice will be higher?</p> <input type="radio" name="choice" id="left" /> <label for="left">dice 1</label><br> <input type="radio" name="choice" id="right" checked /> <label for="right">dice 2</label> <br> <button onclick="roll()"> Roll </button> <script> var guesses_right = 0; var guesses_wrong = 0; function roll(){ choice = ""; if (document.getElementById("left").checked){ choice = "dice1"; } if (document.getElementById("right").checked){ choice = "dice2"; } number1 = Math.floor((Math.random() * 6) + 1); number2 = Math.floor((Math.random() * 6) + 1); link1 = "https://digisoln.com/resources/dice/dice"+number1+".bmp" link2 = "https://digisoln.com/resources/dice/dice"+number2+".bmp" document.getElementById("dice1").src = link1; document.getElementById("dice2").src = link2; } </script>
Question 4: The game you just made in Question #3 is the start of this game (see image below). It uses radio buttons to get a choice from the user. Can you finish the game as pictured here by getting it to tally my right and wrong guesses:
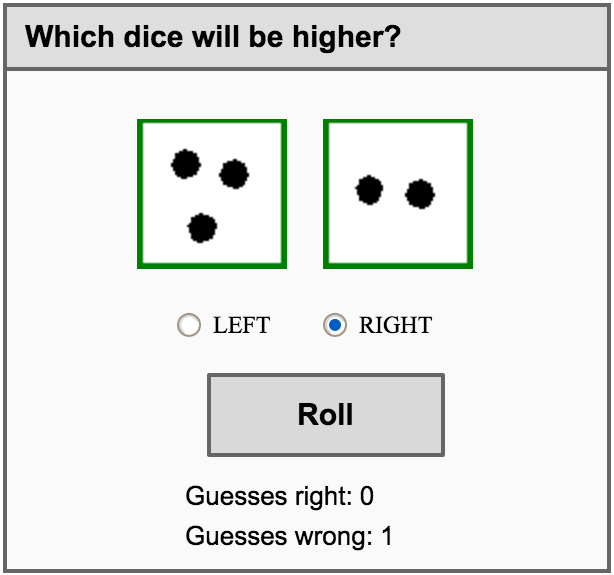
Question 5: Create the following file in VS Code, save it, and launch it in your favourite browser:
Cheat dice.html
<img id="dice1" src="https://digisoln.com/resources/dice/unknown.bmp"> <img id="dice2" src="https://digisoln.com/resources/dice/unknown.bmp"> <select id="cheat"> <option value="double">roll double 6's</option> <option value="snake">roll double 1's</option> </select><br> <input type="checkbox" id="disable_cheats" checked> <label for="disable_cheats"> disable cheats </label> <br> <button onclick="roll()"> Roll </button> <script> function roll(){ cheat = document.getElementById("cheat").value; //listbox disable_cheats = document.getElementById("disable_cheats").checked; //checkbox if (disable_cheats){ // regular dice roll: number1 = Math.floor((Math.random() * 6) + 1); number2 = Math.floor((Math.random() * 6) + 1); link1 = "https://digisoln.com/resources/dice/dice"+number1+".bmp" link2 = "https://digisoln.com/resources/dice/dice"+number2+".bmp" document.getElementById("dice1").src = link1; document.getElementById("dice2").src = link2; } else { // cheat dice roll: if (cheat == "double"){ document.getElementById("dice1").src = "https://digisoln.com/resources/dice/dice6.bmp"; document.getElementById("dice2").src = "https://digisoln.com/resources/dice/dice6.bmp"; } if (cheat == "snake"){ document.getElementById("dice1").src = "https://digisoln.com/resources/dice/dice1.bmp"; document.getElementById("dice2").src = "https://digisoln.com/resources/dice/dice1.bmp"; } } } </script>
Question 6: The game you just made uses a listbox and checkbox to "fix" the dice when i want either a double 6 or a double 1. Your challenge here is to replicate the cheat system in your coin flip game, so that i can choose to enable / disable cheats (using a checkbox), and if i choose to cheat, i can choose to either flip a head or a tail (using a listbox):
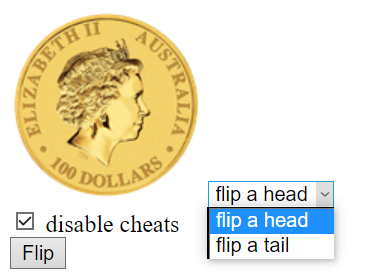