move ball | gml
| Type | Desc |
keyboard events with GML |
keyboard_check(key) | function | check if key is down |
keyboard_check_pressed(key) | function | check if key is pressed |
keyboard_check_released(key) | function | check if key is released |
reading keys with GML |
vk_up | constant | 'virtual keyboard' key for up |
vk_down, vk_space etc | constants | as above |
ord("A") | function | returns the ascii code for the letter "A" |
using keyboard variables with GML |
keyboard_key | variable | stores current key being used |
keyboard_lastkey, keyboard_lastchar, keyboard_string | variables | last key, last character and last 1024 characters (respectively) |
using mouse with GML |
mouse_button | variable | stores current mouse button being pressed |
mouse_x, mouse_y | variables | current axis position of mouse within room |
mouse_check_button(button) | function | check for a button constant |
mb_left, mb_right, mb_middle, mb_none, mb_any | constants | use in the above function (mb_ = mouse button) |
Sprite | Images |
sprBall |  |
Object | Sprite | Properties |
objBall | sprBall | Visible |
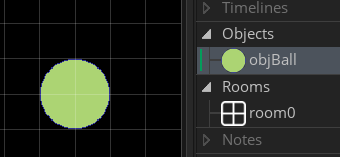
objBall Step
if (keyboard_check(vk_left)){
x = x - 3;
}
if (keyboard_check(vk_right)){
x = x + 3;
}
if (keyboard_check(vk_up)){
y = y - 3;
}
if (keyboard_check(vk_down)){
y = y + 3;
}
Challenge Activities
Replace the code in the
objBall Step event with the following:
1. Interchange functions and keys - how do these changes affect the behaviour of the controls?
objBall Step
if (keyboard_check(ord("A"))){
x = x - 3;
}
if (keyboard_check_pressed(vk_right)){
x = x + 3;
}
if (keyboard_check_released(vk_up)){
y = y - 3;
}
if (keyboard_check(vk_down)){
y = y + 3;
}
2. Working with the keyboard variables using a switch case expression - try this and decide which method you prefer.
objBall Step
switch (keyboard_key) {
case vk_numpad2: y = y + 3; break;
case vk_numpad4: x = x - 3; break;
case vk_numpad6: x = x + 3; break;
case vk_numpad8: y = y - 3; break;
}
3. Try mouse control - can you get this to work without the mouse click (i.e. follow the cursor in the room?)
objBall Step
if (mouse_check_button(mb_any)) {
direction = point_direction(x,y,mouse_x,mouse_y);
speed = 3;
}