pacman | gml
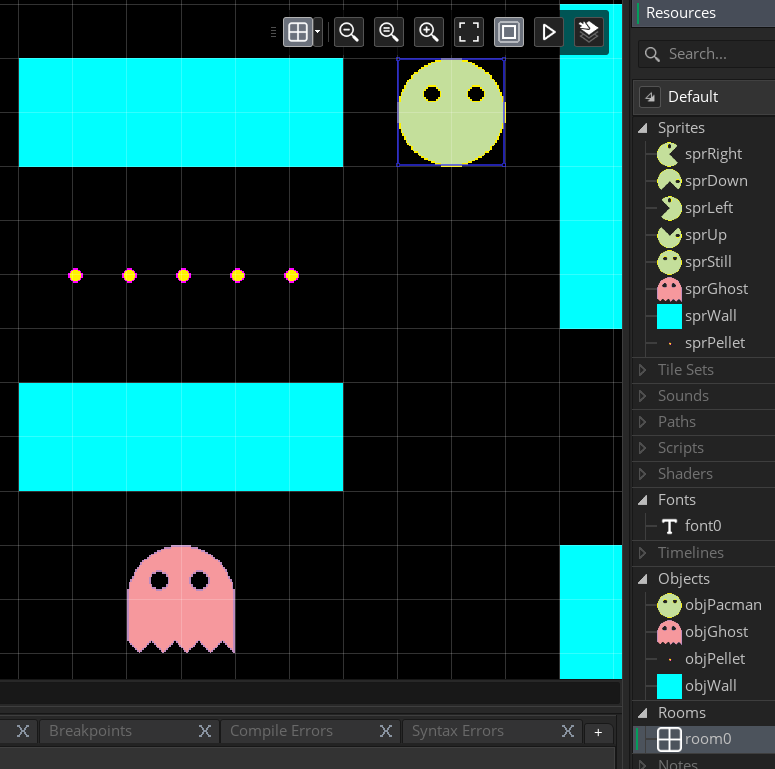
objPacman Create
move_speed = 4; image_speed = 0.25; points = 0; total_pellets = instance_number(objPellet);
objPacman Step
//------------------- MOVEMENT: if (keyboard_check(vk_left) and place_snapped(32, 32)){ sprite_index = sprLeft; motion_set(180,4); } if (keyboard_check(vk_right) and place_snapped(32, 32)){ sprite_index = sprRight; motion_set(0,4); } if (keyboard_check(vk_up) and place_snapped(32, 32)){ sprite_index = sprUp; motion_set(90,4); } if (keyboard_check(vk_down) and place_snapped(32, 32)){ sprite_index = sprDown; motion_set(270,4); } if (keyboard_check(vk_nokey) and place_snapped(32, 32)){ sprite_index = sprStill; motion_set(0,0); } //------------------- BOUNCE OFF WALLS: if(place_meeting(x + hspeed, y + vspeed, objWall)){ x = xprevious; y = yprevious; sprite_index = sprStill; motion_set(0,0); move_snap(32,32); } //------------------- SCORING: var pelletID = instance_place(x,y,objPellet); if pelletID != noone { points += 1; instance_destroy(pelletID); } // if points == total_pellets would have also worked: if (instance_number(objPellet) == 0){ game_restart(); }
objPacman Draw GUI
draw_set_font(font0); draw_set_color(c_fuchsia); draw_text(5,5,"Collected " + string(points)); draw_text(5,25,"Remaining " + string(total_pellets-points));
objGhost Step
if ( //i can only see Pacman within 100px: (distance_to_object(objPacman) <= 100) and //i can't see Pacman behind a wall: (collision_line(x,y,objPacman.x,objPacman.y,objWall,false,true) == noone) and //if i'm on the "grid" and not in the middle of moving: (place_snapped(32, 32)) ){ //if the above conditions are all true, do this: chaseDirection = point_direction(x,y,objPacman.x,objPacman.y); if(chaseDirection > 315) or (chaseDirection < 45) motion_set(0, 2); if(chaseDirection > 45) and (chaseDirection < 135) motion_set(90, 2); if(chaseDirection > 135) and (chaseDirection < 225) motion_set(180, 2); if(chaseDirection > 225) and (chaseDirection < 315) motion_set(270, 2); } else{ if place_snapped(32, 32){ motion_set(0,0); } } //bounce off walls: if(place_meeting(x + hspeed, y + vspeed, objWall)){ move_bounce_all(true); }