moving platforms left & right | gml
Add a new object objMovingRightBlock use the same sprite as the objBlock.. and make the objBlock the parent object. This way the objMovingRightBlock will inherit the properties of the objBlock:
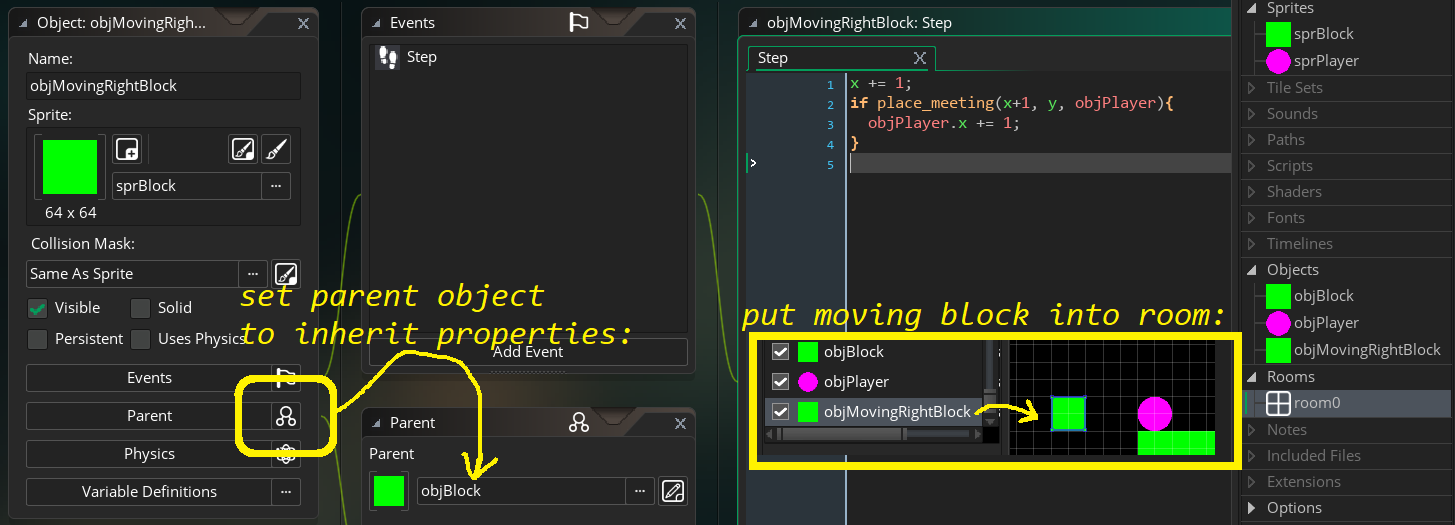
Don't stack the horizontal blocks side by side to make a wider platform. If you do, you may be standing trigger simultaneous collisions with two blocks at once, doubling the speed at which the platform carries you sideways (this will result in you looking like you are travelling twice as fast as the platform under you).
Instead, make a wider platform sprite:
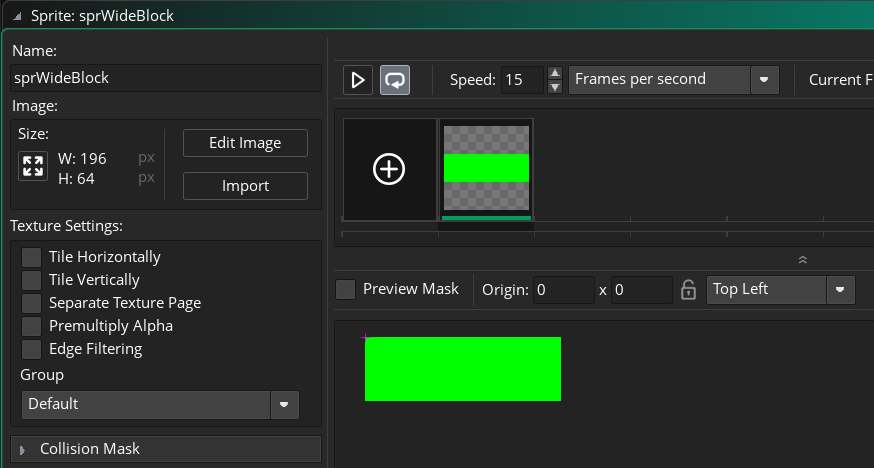
code for step event | gml
Updated 2025: Simply add the following code to STEP event in horizontal moving platform:if (x > (xstart + 200)){ hspeed = -1; } if (x <= xstart){ hspeed = 1; } if place_meeting(x,bbox_top-1,objPlayer){ objPlayer.x += hspeed; }
The code above should be all you need, works in conjunction with Platform Collision Correction code (first lesson)
Challenges
1. Implement the above code2. Add a few moving blocks, adjust the range, move speed and direction (on create), and test them to see if they work.
3. Make a platform only viewable within range.. very difficult! might take some research. You can adjust the image_alpha of an object to make it fade as it disappears from sight (or a torch light beam).