rope swings | gml
Add a new object objRope and set sprite origin Middle Left:
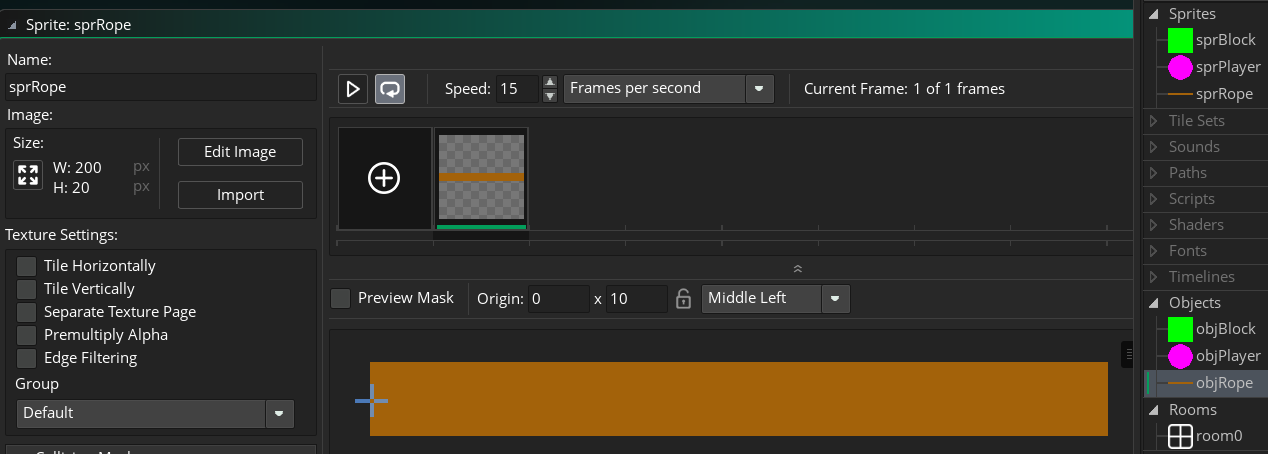
Set up in room with enough room for a decent jump and swing:
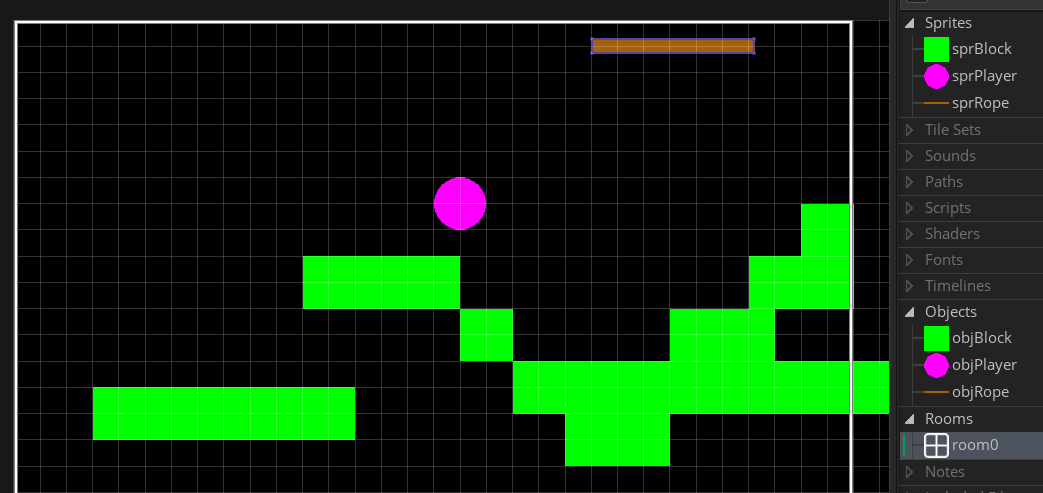
objRope Create
force = 0; direction = 180;
objRope Step
if (direction > 90 && direction < 270){ force = force + 0.25; if (force > 6) force = 6; } else{ force = force - 0.25; if (force < -6) force = -6; } direction += force; image_angle = direction;
objPlayer Create
grav = 1; horiz = 0; vert = 0; move_speed = 7; jump_speed = -15; frict = 0.9; //new variables for adding ropes: on_rope = false; attach_timer = room_speed div 4; //quarter of a second to hold on let_go_timer = 0;
objPlayer Step
// all regular movement applies when i'm not on a rope: if not(on_rope) { if (keyboard_check(vk_right)){ horiz = move_speed; }; if (keyboard_check(vk_left)){ horiz = -move_speed; }; if (keyboard_check(vk_nokey)){ horiz = horiz * frict; }; vert = vert + grav; if (place_meeting(x, y+1, objBlock)) and (keyboard_check(vk_up)) { vert = jump_speed; } if (place_meeting(x+horiz, y, objBlock)) { while (!place_meeting(x+sign(horiz), y, objBlock)) { x = x + sign(horiz); } horiz = 0; } x = x + horiz; if (place_meeting(x, y+vert, objBlock)) { while (!place_meeting(x, y+sign(vert), objBlock)) { y = y + sign(vert); } vert = 0; } y = y + vert; } //---------------------------- SWING ON ROPES: if let_go_timer > 0 let_go_timer -= 1; var rope = instance_place(x,y,objRope); if (rope != noone and let_go_timer < 1) { attach_timer -= 1; if attach_timer < 1 attach_timer = 0; on_rope = true; /*------------------------------------------------------------------------ Because my objPlayer sprite origins are Top Left i am using the following: ------------------------------------------------------------------------*/ x = rope.x+lengthdir_x(rope.sprite_width,rope.direction) - sprite_width/2; y = rope.bbox_bottom-sprite_height/2; /*-------------------------------------------------------------------- If objPlayer sprite origins were Middle Centre then use the following: --------------------------------------------------------------------*/ // x = rope.x+lengthdir_x(rope.sprite_width,rope.direction); // y = rope.bbox_bottom; if attach_timer < 1 { //getting off rope: if keyboard_check(vk_up) { let_go_timer = room_speed div 2; //half a sec to get off rope vert = jump_speed; } if keyboard_check(vk_left) { let_go_timer = room_speed div 2; horiz = -(move_speed); } if keyboard_check(vk_right) { let_go_timer = room_speed div 2; horiz = move_speed; } } } else { on_rope = false; attach_timer = room_speed div 4; }
By scanning for instances of objRope in the player step event (and not the rope step event), all ropes will trigger the on_rope Boolean value to true. This allows for multiple ropes to exist in your level without this happening:
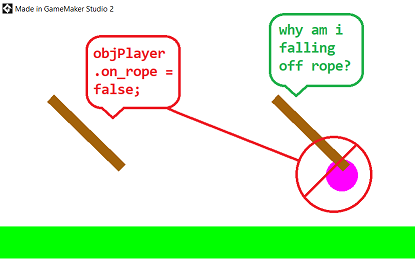
activities
1. Implement the above code2. The rope in the sprite above is 200px long. Try this with a shorter and longer rope.
3. Find an actual rope sprite from the internet to use for this sprite.
4. Can you add some ropes to the previous tutorial you did with up / down / left / right moving platforms? Again, make it increasingly difficult for me (with timed jumps) to get to the end BUT NOT IMPOSSIBLE!