ladders | gml
Add a new object objLadder:
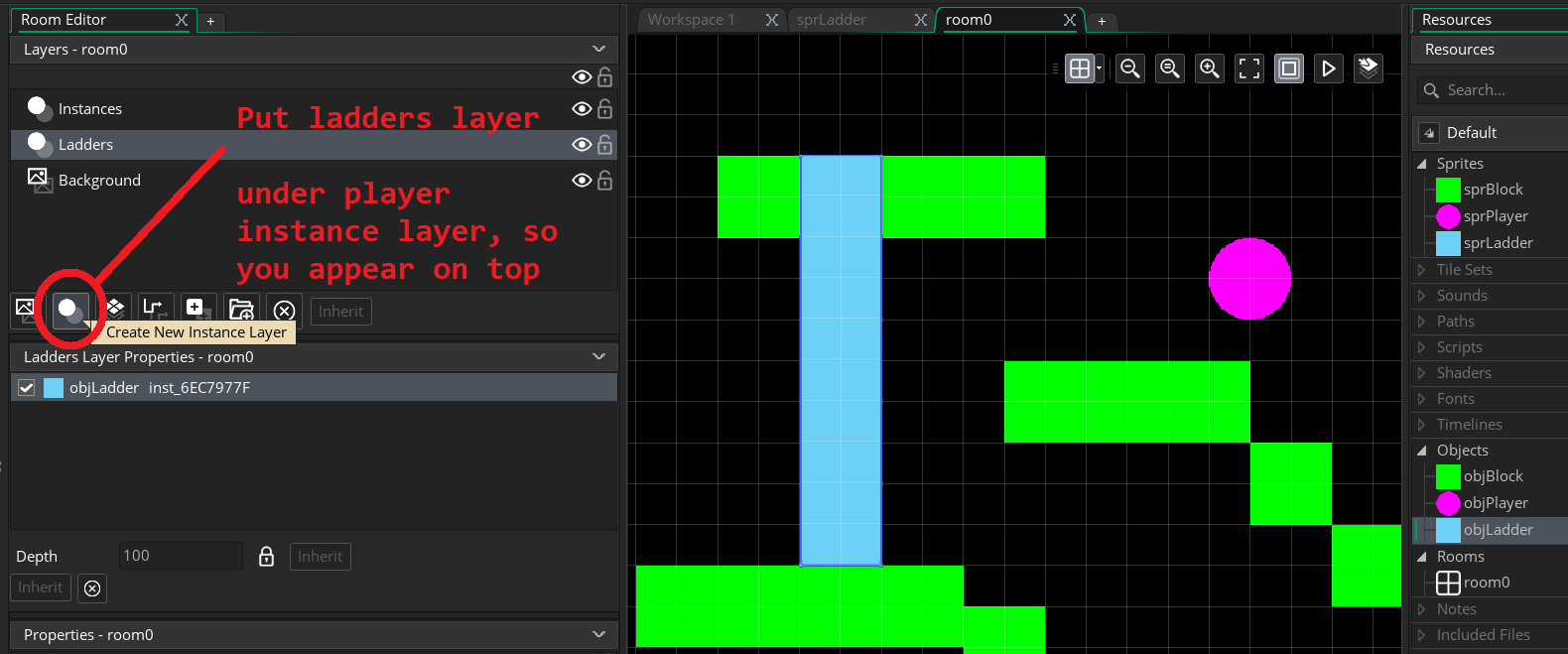
Again, don't stack ladder segments. If you need a big ladder, draw the sprite big:
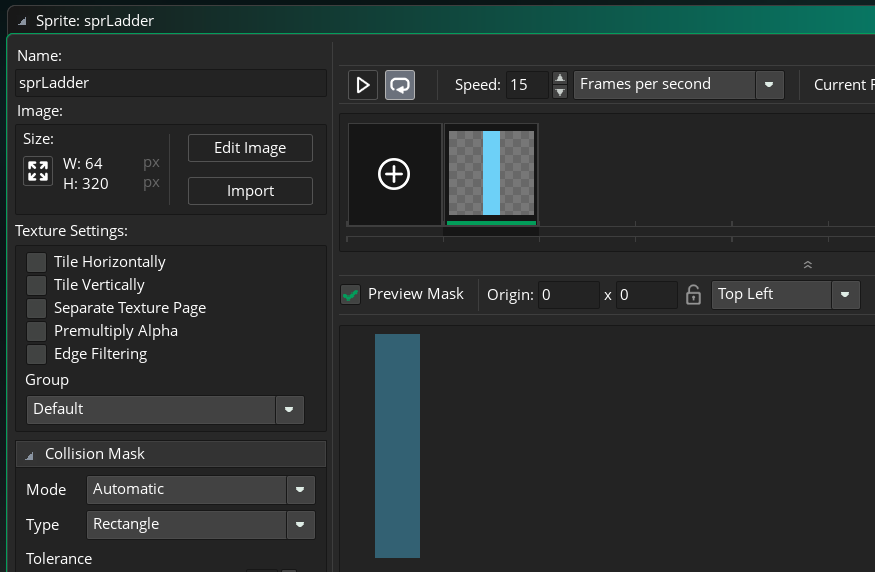
We don't have jumping off top of ladders, nor climbing into ladders from above. So for use now, think about your placement. This works fine and doesnt require jump off top nor climbing down onto from above:
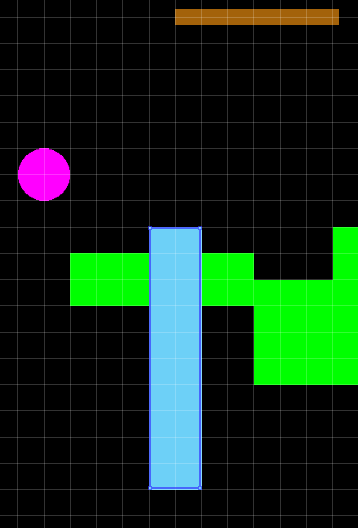
Pro Tip: In case you missed it in the first picture above, put your ladders in a seperate layer below your player sprite, so you appear on top of the ladder and not underneath it:
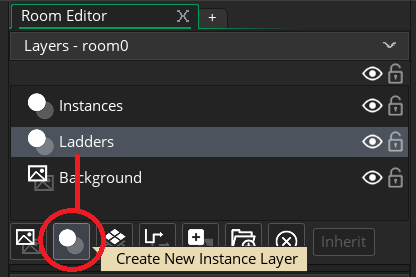
objPlayer Create
grav = 1; horiz = 0; vert = 0; move_speed = 7; jump_speed = -15; frict = 0.9; //new variables for adding ladders: on_ladder = false; ladder_climb_speed = move_speed div 2;
objPlayer Step
// all regular movement applies when i'm not on a ladder: if not(on_ladder){ if (keyboard_check(vk_right)){ horiz = move_speed; }; if (keyboard_check(vk_left)){ horiz = -move_speed; }; if (keyboard_check(vk_nokey)){ horiz = horiz * frict; }; vert = vert + grav; if (place_meeting(x, y+1, objBlock)) and (keyboard_check(vk_up)) { vert = jump_speed; } if (place_meeting(x+horiz, y, objBlock)) { while (!place_meeting(x+sign(horiz), y, objBlock)) { x = x + sign(horiz); } horiz = 0; } x = x + horiz; if (place_meeting(x, y+vert, objBlock)) { while (!place_meeting(x, y+sign(vert), objBlock)) { y = y + sign(vert); } vert = 0; } y = y + vert; } //---------------------------- CLIMB LADDERS: // if i am on a ladder: if place_meeting(x,y,objLadder) and not // ... and im not going to go through the ground // that the ladder has been placed on top of: place_meeting(x,bbox_bottom+1,objBlock) { on_ladder = true; vert = 0; horiz = 0; if keyboard_check(vk_up) y -= ladder_climb_speed; if keyboard_check(vk_down) y += ladder_climb_speed; if keyboard_check(vk_left) x -= ladder_climb_speed; if keyboard_check(vk_right) x += ladder_climb_speed; } else { on_ladder = false; }
Challenges
1. Implement the above code.2. Find a ladder or 'netting' sprite from the internet to use for the ladder sprite.
3. How do ladders work with your existing implementation of ropes, moving and still platforms? Have you encountered and collision stick issues yet?
4. Can you think of any other movement-related elements that would be cool to add to a platform game? Let your teacher know