ai platform enemy | gml
create objEnemy:
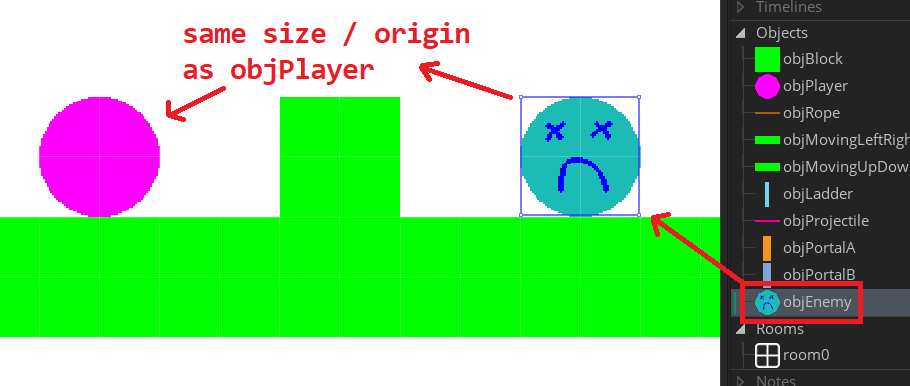
objEnemy Create
grav = 1; horiz = 0; vert = 0; move_speed = 5; jump_speed = -15; frict = 0.9; //simulated inputs and AI variables: aggro_range = 200; left = false; right = false; jump = false;
objEnemy Step
// simulate movement inputs: if (distance_to_object(objPlayer) < aggro_range) { if (objPlayer.x < x){ left = true; } else { left = false; } if (objPlayer.x > x){ right = true; } else { right = false; } //allow some rise in height: if ( objPlayer.y < (y-sprite_height) ){ jump = true; } else { jump = false; } } else { left = false; right = false; jump = false; } if (right){ horiz = move_speed; }; if (left){ horiz = -move_speed; }; if (not(left) and not(right)){ horiz = horiz * frict; }; //friction // add constant force of gravity to our desired vert speed: vert = vert + grav; // only ALLOW jump IF the objBlock is 1 pixel BELOW the enemy, // or in other words, if the enemy is EXACTLY standing on floor: if (place_meeting(x, y+1, objBlock)) and (jump) { vert = jump_speed; } // check for a HORIZONTAL collision: if (place_meeting(x+horiz, y, objBlock)) { // move AS CLOSE AS WE CAN to the HORIZONTAL collision object: // sign() can only return 1, -1, or 0. // sign(-7) == -1 (i.e. negative 1) // sign(7) == 1 (or +1 or positive 1) // sign(0) == 0 while (!place_meeting(x+sign(horiz), y, objBlock)) { x = x + sign(horiz); // move 1 pixel at a time towards object } horiz = 0; // no left right movement on HORIZONTAL collisions } x = x + horiz; // affect calculated horizontal changes by APPLYING to x co-ord // check for a VERTICAL collision: if (place_meeting(x, y+vert, objBlock)) { // move AS CLOSE AS WE CAN to the VERTICAL collision object: while (!place_meeting(x, y+sign(vert), objBlock)) { // remember, sign() can only return 1, -1, or 0: y = y + sign(vert); } vert = 0; // no up down movement on VERTICAL collisions } y = y + vert; // affect calculated vertical changes by APPLYING to x co-ordExtension activities
1. Add your own enemies and adjust thresholds / ranges of speed, distance to aggro etc.
2. Add an additional random jump movement every 2 to 5 seconds
3. How can you modify this code to fit your own ideas or intended purpose for your game?