score health lives | gml
Type | Desc | |
---|---|---|
instance_position(x,y,object) | function | almost identical to instance_place(x,y,object), however instance_position checks for a collision within a specific pixel, whereas instance_place checks for a collision within a collision mask. Both functions return the instance_id of the object collision that we can do stuff with. |
object_get_name(object_index) | function | we are using this as a bit of a hack to return the string name of the object we clicked on. basically can tell us which object is running the current piece of code being executed. |
other | keyword | opposite of self, used in a with block, can refer back to the original calling object |
draw_healthbar(lots of arguments) | function | draws a healthbar, when you look at the arguments, be aware (x1, y1) == (top left corner) and (x2, y2) == (bottom right corner) |
draw_set_font(font) | function | if you want your game published to HTML5, and you plan on using draw_text() , then you have to specify a font for this to work.. so it is a good habit to get into declaring your fonts explicitly. |
Sprite | Images |
---|---|
sprPlusOne | ![]() |
sprMinusTwo | ![]() |
sprLifeIcon | ![]() |
Object | Sprite | Properties |
---|---|---|
objPlusOne | sprPlusOne | Visible |
objMinusTwo | sprMinusTwo | Visible |
objPlayer | no sprite |
Font:
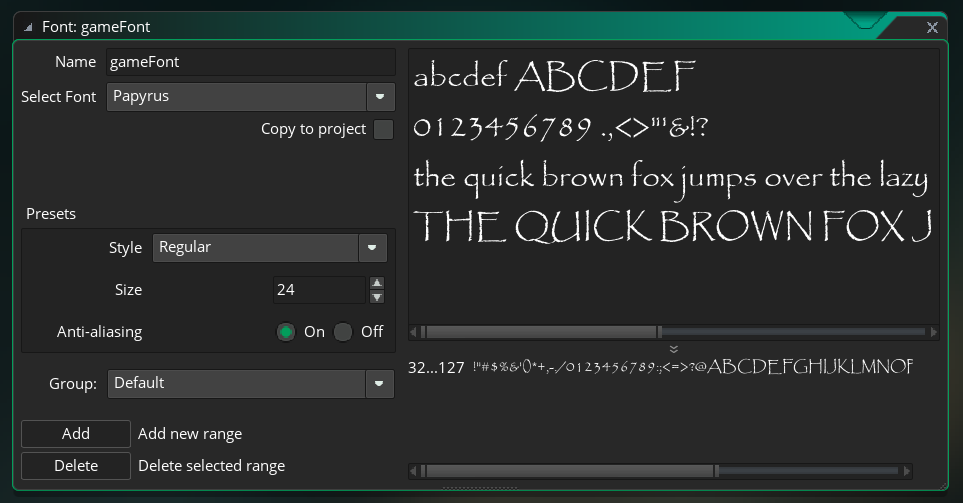
Room layout:
objPlayer Create
myHealth = 100; myLives = 3; myScore = 0;
objPlayer Step
//if a button is clicked: if (mouse_check_button_pressed(mb_left)) { //'with' the other object (if there is one): with(instance_position(mouse_x, mouse_y, all)){ //find out if a button object was under the mouse: switch(object_get_name(object_index)){ //since we are now 'with' the button reference, //using 'other' will refer back to objPlayer: case "objPlusOne": other.myHealth += 1; break; case "objMinusTwo": other.myHealth -=2; break; } } //one point just for clicking somewhere on screen: myScore += 1; } if (myHealth < 1){ myLives -= 1; myHealth = 100; }
objPlayer Draw GUI
//***RECTANGULAR HEALTH BAR*** // draw_healthbar(x1, y1, x2, y2, // amount, background_colour, min_colour, max_colour, // direction, show_background_colour, show_border); draw_healthbar(x-50, y-10, x+50, y-5, myHealth, c_black, c_red, c_lime, 0, true, true) for(i = 0; i < myLives; i++){ draw_sprite(sprLifeIcon, -1, i*100, 0) } draw_set_font(gameFont); draw_set_color(c_white); draw_text(x,y,myScore);
Challenge: use these skills to add lives, score and health to your previous games