terrain generation | gml
Create a 64 x 64 sprite sprBlock and assign to objBlock, but DO NOT place any blocks into room.
We will generate our terrain programmatically using the room Creation Code:
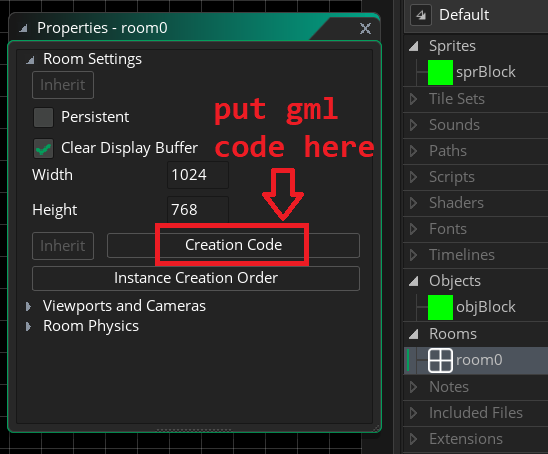
Default room dimensions of 1024 x 768 means we have a grid of 16x12 blocks:
1024 / 64 = 16, 768 / 64 = 12
This means we have an "arbitrary space" grid of 16 spaces horizontally and 12 spaces vertically to work with:
room0 Creation Code
//------------------------ SPECIFICATIONS -----------: //max / min height (in blocks): maxHeight = 9; minHeight = 3; //to calculate height and width of grid, got to //spawn an instance of the block first: temp = instance_create_layer(0,0,"Instances",objBlock); blockHeight = objBlock.sprite_height; blockWidth = objBlock.sprite_width; gridHeight = room_height / blockHeight; gridWidth = room_width / blockWidth; instance_destroy(temp); //---------------------------------------------------- // TO CREATE TERRAIN: //1. Set co-ords for our first block: randomize(); xx = 0; yy = irandom_range(gridHeight - maxHeight, gridHeight - minHeight); //2. Moving across the screen... for(columnToFill = xx; columnToFill <= gridWidth; columnToFill += 1){ //3. .. work out up one, same height or down one for each column .. upSameDown = irandom_range(1,3); //1 up, 2 same height, 3 down switch(upSameDown){ case 1: if(yy > (gridHeight - maxHeight))yy -= 1; break; case 2: break; //do nothing - same height! case 3: if(yy < (gridHeight - minHeight))yy += 1; break; } //4. .. and fill each column to the ground: for(columnPos = yy; columnPos <= gridHeight; columnPos += 1){ instance_create_layer(columnToFill * blockWidth, columnPos * blockHeight, "Instances", objBlock); } }