top down player | gml
diagonal movement problem
In poorly designed 2D games, where the camera is viewing the player from a top-down angle, players that move one "unit" right, and one "unit" up, may also move that distance diagonally if both up and right are pushed simulatenously.
However, this results in the player moving diagonally at a rate of 1.414 times faster than they would move right or left:
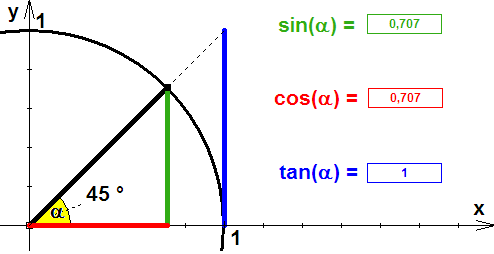
To fix this, diagonal (i.e. simulataneous) keypresses should be checked for, and if this is the case, the speeds should be "normalised", so that the speed right or up is equal to the speed diagonally. You could do this by multiplying the diagonal speed by 0.7ish.. but there are better ways.
Sprite | Images |
---|---|
sprTopDownPlayer | ![]() |
sprLazer | ![]() |
Middle-centre the origins for both sprTopDownPlayer and sprLazer.
Object | Sprite | Properties |
---|---|---|
objTopDownPlayer | sprTopDownPlayer | Visible |
objLazer | sprLazer | Visible |
Simply place the objTopDownPlayer somewhere in the room but not the objLazer - we will spawn that in programmatically using GML.
objTopDownPlayer Step
movespeed = 5; key_up = -keyboard_check(ord("W")); key_down = keyboard_check(ord("S")); key_right = keyboard_check(ord("D")); key_left = -keyboard_check(ord("A")); move_x = key_left + key_right; move_y = key_up + key_down; //Compensate for diagonal input: if (move_x != 0 and move_y != 0){ //diagonal speed multiplyer - diagonal = 0.707; }else{ //regular speed multiplyer - diagonal = 1; } hspeed = move_x * movespeed * diagonal; vspeed = move_y * movespeed * diagonal; directionToCursor = point_direction(x,y,mouse_x,mouse_y); image_angle = directionToCursor; if mouse_check_button(mb_any){ lazer = instance_create_depth(x,y,0,objLazer); lazer.speed = 10; lazer.direction = directionToCursor; lazer.image_angle = directionToCursor; }
Challenge: How can you improve this game?