Catching errors
debugging.py
from flask import * app = Flask(__name__) @app.route("/") def main(): try: x = 0 / 0 return "This code runs, but zero division doesn't." except Exception as error: return repr(error) app.run(debug=True)
invalidsyntax.py
### THIS CONTAINS A DELIBERATE ERROR ### AND WILL NOT RUN IN ITS CURRENT STATE: from flask import * app = Flask(__name__) @app.route("/") def main(): Lorem ipsum dolor sit amet... # ^^ PRODUCES FATAL ERROR - WILL NOT RUN! # YOU NEED A BATCH FILE TO SEE ERROR REPORT: # cmd /k python invalidsyntax.py # OR IF THAT DOESN'T WORK: # cmd /k py invalidsyntax.py # SAVE AS BATCH FILE AS: run.bat app.run(debug=True)The first example above returns the error via the browser window:
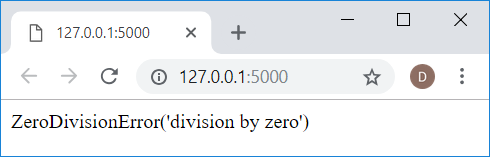
You can put potential code breaking script in
try
/ except
blocks in order to discover (or feedback) what is causing the error. There are specific errors you can target in order to avoid errors 'hiding' behind other errors (e.g. ValueError) which is preferable, but the approach above will suffice for this course.The second approach won't run from IDLE (or your prefferred IDE), although, if you are launching the script from CMD (or terminal on MAC), then the Command Prompt window will actually close before you get a chance to see the error preventing the app from launching.
As a workaround solution, create a .bat file (using notepad) and add the following line to the batch command:
cmd /k python invalidsyntax.py
When you launch the Flask application via the batch file, the command prompt window will not close (instantly), thanks to the /k
: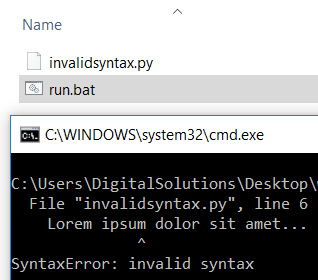