Image Processing
To install Pillow:pip3 install pillow
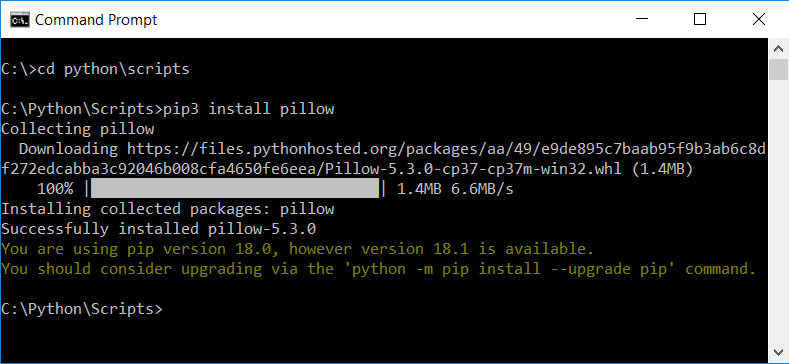
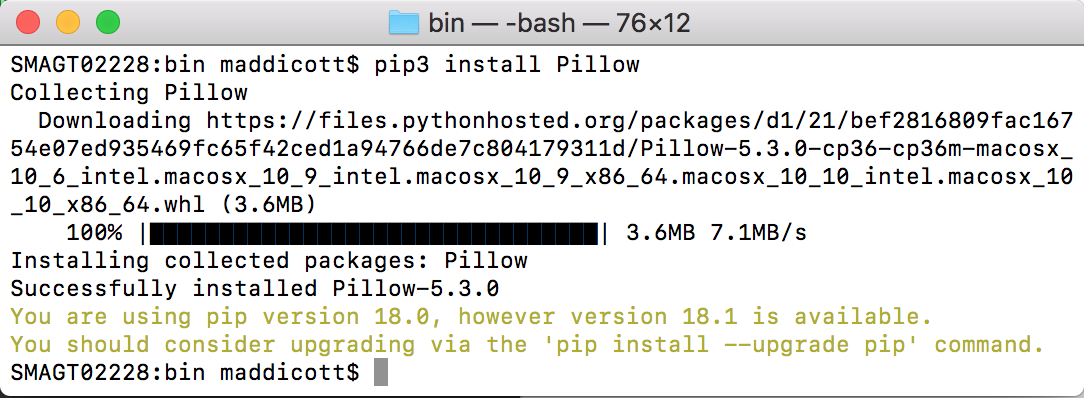
simple_pillow.py
from PIL import Image import urllib.request try: #get an original image: url = "http://www.digisoln.com/teachers/there-is-no-cloud.png" g = urllib.request.urlopen(url) with open('cloud.png', 'b+w') as f: f.write(g.read()) #load both images into memory for comparison: original = Image.open("cloud.png") thumb = Image.open("cloud.png") #edit thumbnail image data: thumb.thumbnail((128, 128)) #^^ 128x128 is max dimensions for thumb, not the scale size! #this will keep the thumbs proportionality locked (not skewed). #this will also NOT enlarge an image thumb.save("th_cloud.png") #compare both images: print("Original: ", original.format, original.size, original.mode) print("Thumbnail: ", thumb.format, thumb.size, thumb.mode) except Exception as error: print(repr(error))
extended_pillow.py
from PIL import Image, ImageFilter import urllib.request try: #get an original image: url = "http://digisoln.com/teachers/stacked.jpg" g = urllib.request.urlopen(url) with open('stacked.jpg', 'b+w') as f: f.write(g.read()) #load image into memory: imagedata = Image.open("stacked.jpg") #Filters available in Pillow: #BLUR, CONTOUR, DETAIL, EDGE_ENHANCE, EDGE_ENHANCE_MORE #EMBOSS, FIND_EDGES, SMOOTH, SMOOTH_MORE, SHARPEN: imagedata = imagedata.filter(ImageFilter.CONTOUR) #resize and compress the image: width, height = imagedata.size newsize = (width, int(round(height / 2))) imagedata = imagedata.resize(newsize, Image.ANTIALIAS) imagedata.save("stacked_changed.jpg", quality=5) #5% imagedata.show() #this will show a temporary display except Exception as error: print(repr(error))