Understanding some basics
Installing Flask
You need to install Python first. If you didn't change the default installation options of Python, then it is likely your Python folder will be found in C:\Users\<username>\AppData\Local\Programs\Python\Python38-32\Scripts
HTTP Methods
The two most common HTTP methods are: GET and POST. GET requests can be cached, bookmarked, and should not be used for sensitive data. POST requests are the opposite and have no restrictions on data length. Most requests you use will be GET requests, except if you are sending data to a server to create or update a resource (e.g., database).
Decorators
A decorator @ is simply a pretty way to take a function, add some functionality to it, then return it:
decorators.py
### WRAP YOUR BURGER FILLING! ### def burger(filling): print("Top piece of bread") print(filling()) print("Bottom piece of bread") def tofu(): return "Tofu" tofu = burger(tofu) ### <-- without decorator @burger ### <-- with decorator def meat(): return "Meat"
Decorators are used in the Flask examples so when you see this:
from flask import *
app = Flask("My Application")
def home():
return "Home page"
home = app.route("/", methods=["GET","POST"])(home)
app.run(debug=True, host="127.0.0.1", port=5000)
it is the same as this:
from flask import *
app = Flask("My Application")
@app.route("/", methods=["GET","POST"])
def home():
return "Home page"
app.run(debug=True, host="127.0.0.1", port=5000)
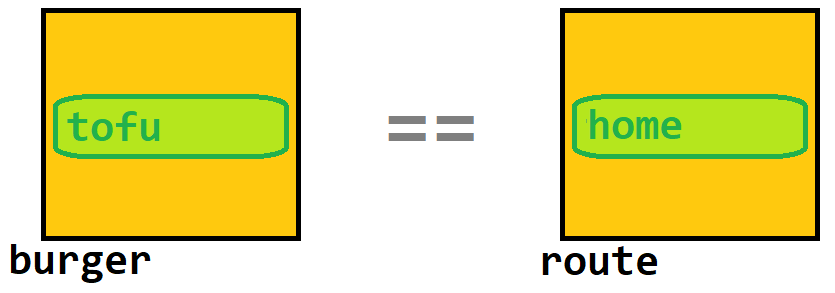