web sockets
A websocket is a persistent connection between your browser and the server that messages can be sent across. Using a websocket connection allows a server to "push" notifications to the client seamlessly, rather than the client polling for updates: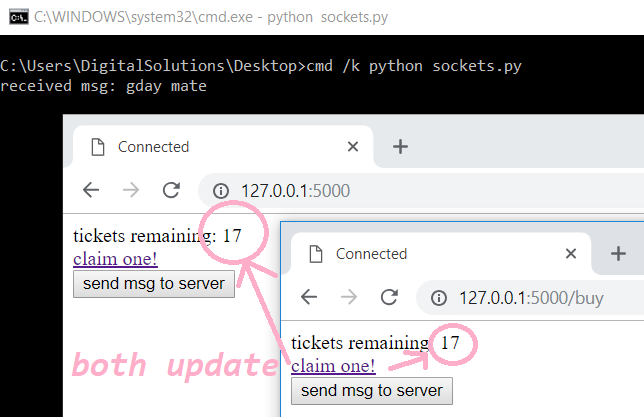
sockets.py
from flask import Flask, render_template_string from flask_socketio import SocketIO, send, emit app = Flask(__name__) socketio = SocketIO(app) tickets = 20 output = ''' <script src="https://cdn.socket.io/socket.io-1.0.0.js"></script> <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script> <script> var socket = io.connect("http://127.0.0.1:5000"); socket.on('connect', function() { document.title = "Connected"; }); //************* TICKET TAKEN BY ANOTHER USER: socket.on('available', function(tickets) { document.getElementById("ticks").innerHTML = tickets; }); //************* SEND A MESSAGE TO THE SERVER: $(document).ready( function() { $('.gday' ).click(function() { socket.emit("ClientToServer", "gday mate"); }); }); </script> tickets remaining: <span id='ticks'>{{ tickets }}</span><br> <a href='/buy'>claim one!</a><br> <button class='gday'>send msg to server</button><br> ''' @app.route('/') def index(): global tickets return render_template_string(output, tickets = tickets) @app.route('/buy') def ticketSold(): global tickets tickets -= 1 socketio.emit('available',tickets) return render_template_string(output, tickets = tickets) @socketio.on('ClientToServer') def handle(msg): print('received msg: ' + msg) socketio.run(app)
Installing Web Sockets
To install sockets on Windows, first install Microsoft Visual C++ 14.0 (found in Microsoft Visual C++ Build Tools):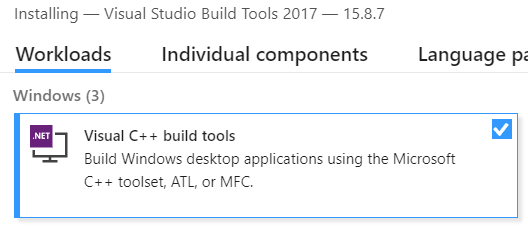
Then install flask socketio:
pip3 install flask-socketio
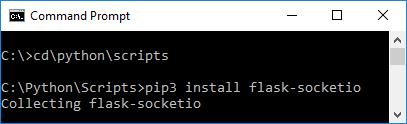
Allow connections through Windows firewall:
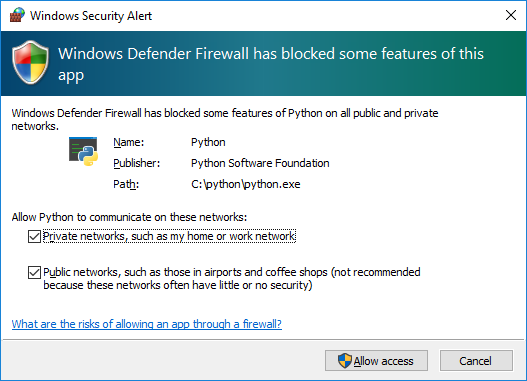
To install sockets on Mac:

... which will trigger the xcode developer tools download ...
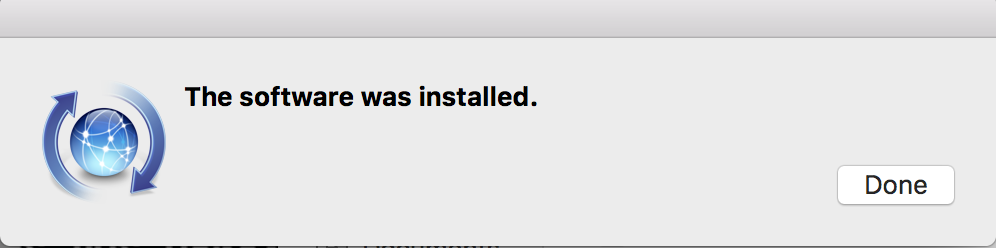
once xcode developer tools have installed, reinstall gevent if it didn't finish properly before:

finally, pip3 install flask-socketio, as above:
