Recording chosen books
books_receipt.py
import sqlite3 db = sqlite3.connect('bookshop.db') result = db.cursor().execute("SELECT * FROM books").fetchall() db.close() my_books = [] menu = """Bookshop menu. 1: Choose book 2: View my books 3: Exit >> """ menu_choice = int(input(menu)) while menu_choice != 3: if menu_choice == 1: n = len(result) i = 0 while i < n: print(i, result[i][1]) #e.g.: 10 The Gruffalo i = i + 1 choice = int(input("Enter book num >> ")) my_books.append(result[choice]) if menu_choice == 2: print(my_books) menu_choice = int(input(menu))How this works:
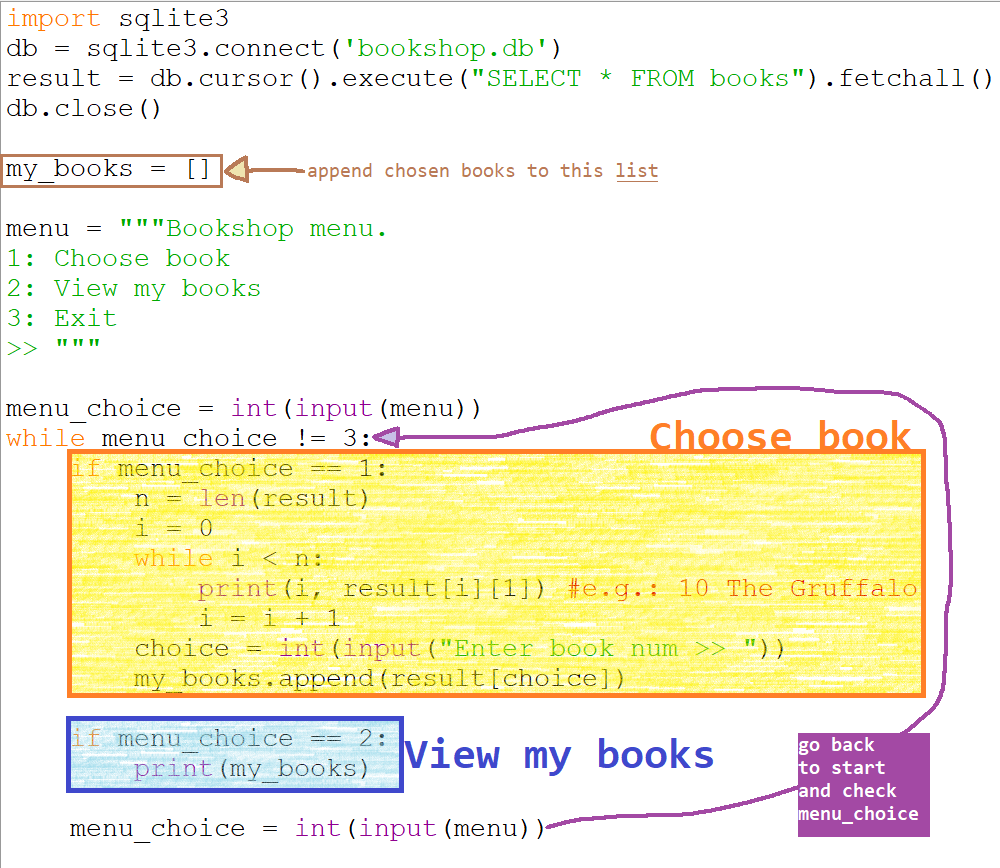
- Run the code above and purchase some books.
- Explain in your own words how this line of code works: my_books.append(result[choice])
- When i chose to view the books I have chosen (currently choice number 2 in this program), make this program only show the titles of the books I have chosen. Hint: You will need another loop, and this:
n = len(my_books)
- When i exit the program, print out the my_books list in a presentable format, that shoes me all the titles, authors and prices, total price, total credit and credit remaining. It might look like this:
Where's Wally by Martin Hanford. Price: $20.8 The Gruffalo by Donaldson, Julia. Price: $21.95 Total price: $42.75 Total credit: $50 Credit remaining: $7.25
- Could the n = len(result) variable (that stores the number of tuples in the books dataset) be calculated at launch of this program (i.e. when i first run the SQL query)? Is there any need for this variable to be recalculated every time I choose choice number 1? Test and discuss.
Core Activities:
Extension Activities: