Inserting (adding) another book
insert_book.py
import sqlite3 db = sqlite3.connect('bookshop.db') new_title = input("Title: ") new_category = input("Category: ") new_description = input("Description: ") new_price = float(input("Price: ")) new_author = input("Author: ") db.cursor().execute(""" INSERT INTO books (title, category, description, price, author) VALUES (?, ?, ?, ?, ?) """, (new_title, new_category, new_description, new_price, new_author) ) db.commit() result = db.cursor().execute("SELECT * FROM books").fetchall() db.close() print(result)
Use the SQL placeholders (?) to inject variables into SQL statements:
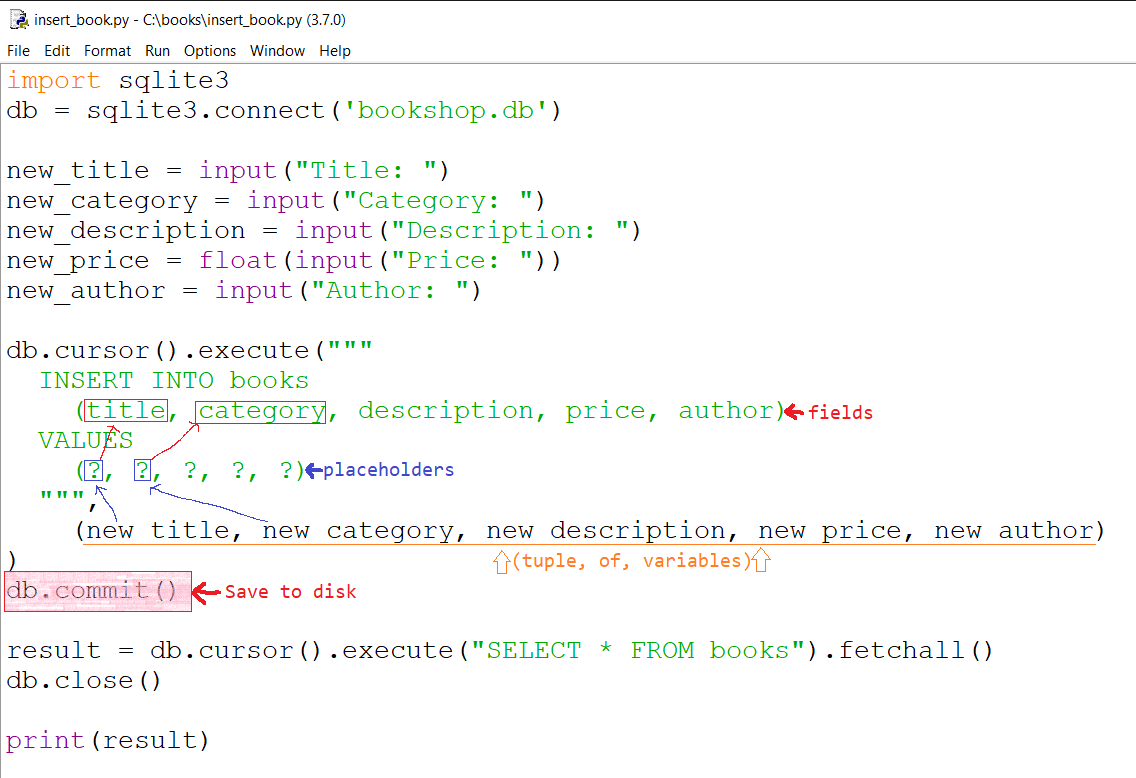