Inputs
create an object called "gameController", and drag and drop it into your room (it has no sprite):
add a top-down (aerial view) car object to your room. make sure you centre the origin:
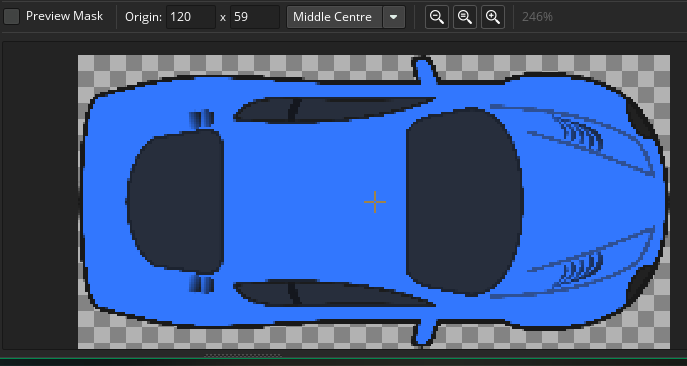
add all of the following code blocks to the gameController as shown:
Create and Step events:
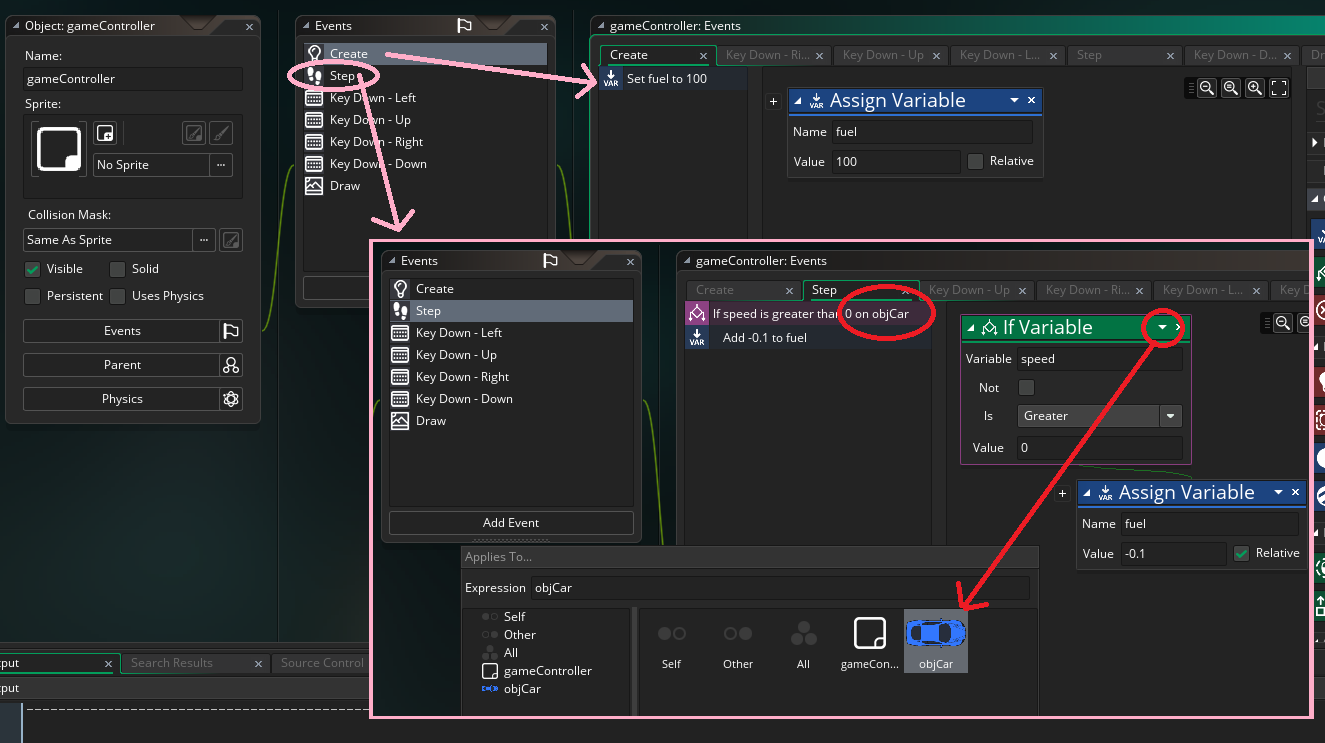
Key Down - Up:
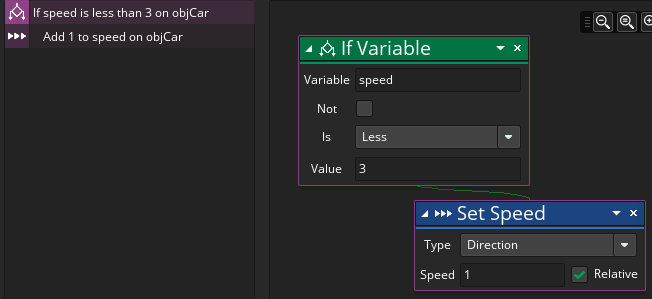
Key Down - Right:
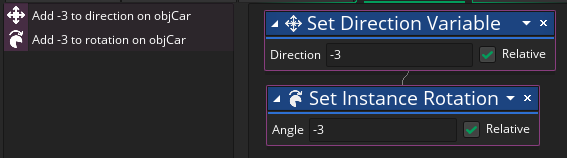
^^for left just make the values positive, also for down (stopping) set the speed to 0
Draw:
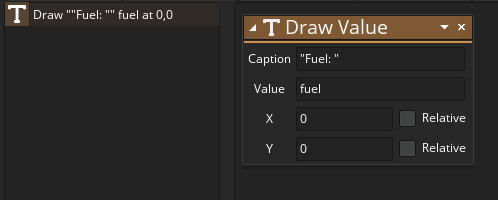
put an object called "gameController" (it has no sprite) into an empty room (see the first image at the top of this page). put the following GML on the gameController to test how keyboard and mouse input works:
Draw event:
draw_text(5, 0, "Keyboard last key: " + string(keyboard_lastkey)); draw_text(5, 25, "Current key: " + string(keyboard_key)); draw_text(5, 50, "Keyboard last char: " + keyboard_lastchar); draw_text(5, 75, "Last 1024 chars: " + keyboard_string); draw_text(5, 100, "**Space bar tests as follows:**"); if (keyboard_check(vk_space)) draw_text(5, 125, "Space is currently down"); if (keyboard_check_pressed(vk_space)) draw_text(5, 150, "Space was pressed once during the last step"); if (keyboard_check_released(vk_space)) draw_text(5, 175, "Space was released once during the last step"); if (keyboard_check_direct(vk_space)) draw_text(5, 200, "Space is currently down even if i am on another program"); draw_text(5, 225, "**Mouse tests as follows:**"); if (mouse_check_button(mb_any)) draw_text(5, 250, "Button down detected."); if (mouse_button == mb_none) draw_text(205, 250, "No buttons, or button released detected."); switch(mouse_button){ case mb_left: draw_text(5, 275, "Left mouse button down"); break; case mb_right: draw_text(5, 275, "Right mouse button down"); break; case mb_middle: draw_text(5, 275, "Middle mouse button down"); break; } draw_text(5, 300, "mouse_x: " + string(mouse_x)); draw_text(5, 325, "mouse_y: " + string(mouse_y));
putting it together
add the car as was done in the second step at the top of this page... then all of the following GML goes into the gameController object (although you could modify it easily to fit into the car object)
Create event:
fuel = 100;
Draw event:
draw_text(0,0,"Fuel remaining: " + string(fuel))
Step event:
if (keyboard_check(vk_left)){ objCar.direction = objCar.direction + 3 objCar.image_angle = objCar.image_angle + 3 } if (keyboard_check(vk_right)){ objCar.direction = objCar.direction - 3 objCar.image_angle = objCar.image_angle - 3 } if (keyboard_check(vk_up)){ if(objCar.speed < 3) { objCar.speed = objCar.speed + 1 } } if (keyboard_check(vk_down)){ if(objCar.speed > -1) { objCar.speed = objCar.speed - 1 } } switch(objCar.speed){ case 1: fuel = fuel - 0.01; break; case 2: fuel = fuel - 0.05; break; case 3: fuel = fuel - 0.1; break; }