GML - Animating Platformer Player
This activitiy continues on from the previous Platform Collision Basics activity
Sprite | Images |
---|---|
sprStill | ![]() centre every player sprite you import shown below: ![]() |
sprWalk |
![]() ![]() ![]() ![]() ![]() ![]() ![]() or as a zip (remember to keep centering all player sprites): walk.zip |
sprJumpUp | ![]() |
sprLanding | ![]() |
sprBlock | ![]() |
Object | Sprite | Properties |
---|---|---|
objPlayer | sprStill | Visible |
objBlock | sprBlock | Visible |
Use the room layout from the previous Platform Collision Basics example
But this time, (A) replace the player sprite, (B) change the background colour so you can see the sprite:
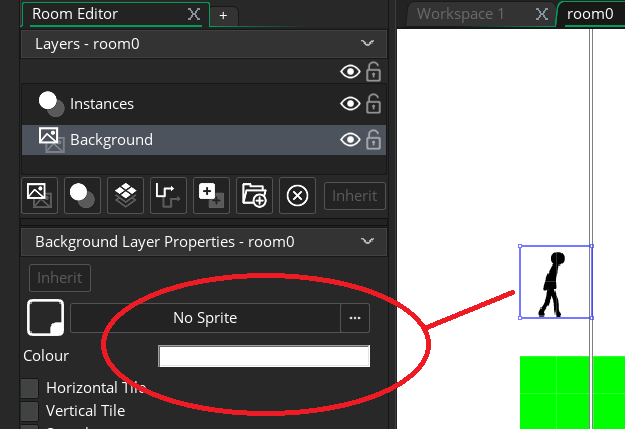
grav = 1; //our gravity horiz = 0; //our desired horizontal speed vert = 0; //our desired vertical speed move_speed = 5; jump_speed = -12; frict = 0.95; facing = 1; //start facing right jumped = false; //controls jump animations
// left and right movement inputs AND animations: if (keyboard_check(vk_right)){ horiz = move_speed; facing = 1; }; if (keyboard_check(vk_left)){ horiz = -move_speed; facing = -1; }; if (keyboard_check(vk_left) or keyboard_check(vk_right) and not jumped) { sprite_index = sprWalk; } if (keyboard_check(vk_nokey)){ horiz = horiz * frict; //friction if (abs(horiz) < 1 and abs(vert) < 1) { sprite_index = sprStill }; }; // add constant force of gravity to our desired vert speed: vert = vert + grav; // only ALLOW jump IF the objBlock is 1 pixel BELOW the player, // or in other words, if the player is EXACTLY standing on floor: if (place_meeting(x, y+1, objBlock)) and (keyboard_check(vk_up)) { vert = jump_speed; jumped = true;} // jump animations: if (jumped) { if (vert > 0) { sprite_index = sprJumpUp; } else { sprite_index = sprLanding; } if (place_meeting(x, y+1, objBlock)) { jumped = false; } } // check for a HORIZONTAL collision: if (place_meeting(x+horiz, y, objBlock)) { // move AS CLOSE AS WE CAN to the HORIZONTAL collision object: // sign() can only return 1, -1, or 0. // sign(-7) == -1 (i.e. negative 1) // sign(7) == 1 (or +1 or positive 1) // sign(0) == 0 while (!place_meeting(x+sign(horiz), y, objBlock)) { x = x + sign(horiz); // move 1 pixel at a time towards object } horiz = 0; // no left right movement on HORIZONTAL collisions } // we have worked out our horizontal speed, now APPLY the change: x = x + horiz; // check for a VERTICAL collision: if (place_meeting(x, y+vert, objBlock)) { // move AS CLOSE AS WE CAN to the VERTICAL collision object: while (!place_meeting(x, y+sign(vert), objBlock)) { // remember, sign() can only return 1, -1, or 0: y = y + sign(vert); // // move 1 pixel at a time towards object } vert = 0; // no up down movement on VERTICAL collisions } // we have worked out our vertical speed, now APPLY the change: y = y + vert;
draw_sprite_ext( sprite_index, image_index, x, y, facing, //instead of image_xscale image_yscale, image_angle, image_blend, image_alpha );
image_speed = 0.5;
We are getting lots of image stick that we will fix in the next activity