GPS Tracker
Setup App
if you dont know how to do this look back into past tutorials:
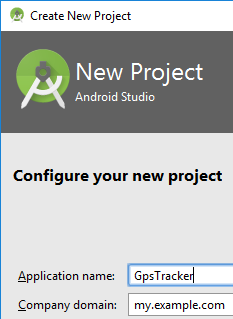
Layout Editor
two TextView objects, named txtLongitude and txtLatitude:
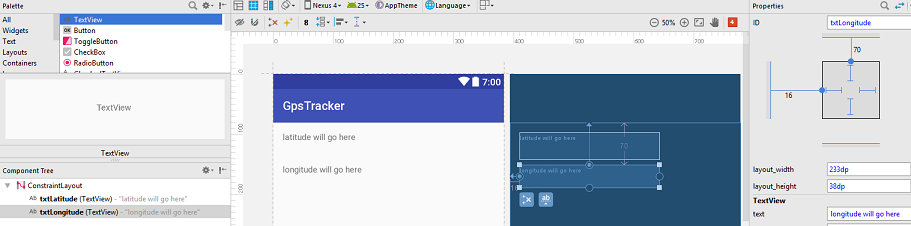
MainActivity Code
goes in MainActivity.java:package com.example.my.gpstracker; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; //~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ //for global information about application environment: import android.content.Context; //for getting permissions at runtime: import android.Manifest; import android.support.v4.app.ActivityCompat; import android.support.v4.content.ContextCompat; import android.content.pm.PackageManager; //for geographic information: import android.location.LocationListener; import android.location.LocationManager; import android.location.Location; //for the UI: import android.widget.TextView; //to make our lives easier when debugging: import android.util.Log; //~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ public class MainActivity extends AppCompatActivity { //~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ //Variables: protected Context myContext; protected LocationManager manageMyGPS; protected LocationListener listenToMyGPS; private TextView myLatitude; private TextView myLongitude; private int ACCESS_COARSE_LOCATION_permission; private int ACCESS_FINE_LOCATION_permission; //~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); //~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ //bind UI objects and current context permissions: myLatitude = (TextView) findViewById(R.id.htmlLatitude); myLongitude = (TextView) findViewById(R.id.htmlLongitude); ACCESS_COARSE_LOCATION_permission = ContextCompat.checkSelfPermission(this, Manifest.permission.ACCESS_COARSE_LOCATION); ACCESS_FINE_LOCATION_permission = ContextCompat.checkSelfPermission(this, Manifest.permission.ACCESS_FINE_LOCATION); //~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ //define (but not commence yet) our GPS listener behaviour: listenToMyGPS = new LocationListener() { public void onStatusChanged(String provider, int status, Bundle extras) {} public void onProviderEnabled(String provider) {} public void onProviderDisabled(String provider) {} public void onLocationChanged(Location location) { myLongitude.setText(String.valueOf(location.getLongitude())); myLatitude.setText(String.valueOf(location.getLatitude())); } }; //~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ //check for permissions at runtime: if(ACCESS_FINE_LOCATION_permission == PackageManager.PERMISSION_DENIED){ ActivityCompat.requestPermissions(this, new String[]{Manifest.permission.ACCESS_FINE_LOCATION}, 1337); } else { if (ACCESS_COARSE_LOCATION_permission == PackageManager.PERMISSION_DENIED) { ActivityCompat.requestPermissions(this, new String[]{Manifest.permission.ACCESS_COARSE_LOCATION}, 9999); } else{ goGPS(); } } }//end of onCreate() //***************************************** //UTILITY METHODS: //on first run, if we have allow permission, this is the "callback" event to keep working: @Override public void onRequestPermissionsResult(int requestCode, String permissions[], int[] grantResults) { goGPS(); } //~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ //this method invokes our GPS listener: public void goGPS(){ //ready gps: manageMyGPS = (LocationManager) getSystemService(myContext.LOCATION_SERVICE); //got to make sure we have permission: try { //get gps co-ords: manageMyGPS.requestLocationUpdates(LocationManager.GPS_PROVIDER, 0, 0, listenToMyGPS); } catch (SecurityException e) { //no permissions granted yet (or something else went wrong): Log.i("requestLocationUpdates", "rekt bruh: " + e.toString()); } } }
AndroidManifest Code
add these lines to the AndroidManifest.xml after the final </application> tag:<!--annoyingly we will still have to check for these permissions at runtime --> <uses-permission android:name="android.permission.ACCESS_FINE_LOCATION"></uses-permission> <uses-permission android:name="android.permission.ACCESS_COARSE_LOCATION"></uses-permission>
so my completed AndroidManifest.xml looks like this, although yours may look slightly different:
<?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" package="com.example.my.gpstracker"> <application android:allowBackup="true" android:icon="@mipmap/ic_launcher" android:label="@string/app_name" android:roundIcon="@mipmap/ic_launcher_round" android:supportsRtl="true" android:theme="@style/AppTheme"> <activity android:name=".MainActivity"> <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> </application> <!--annoyingly we will still have to check for these permissions at runtime --> <uses-permission android:name="android.permission.ACCESS_FINE_LOCATION"></uses-permission> <uses-permission android:name="android.permission.ACCESS_COARSE_LOCATION"></uses-permission> </manifest>
Run
should ask for permissions first time, then launch goGPS() event:
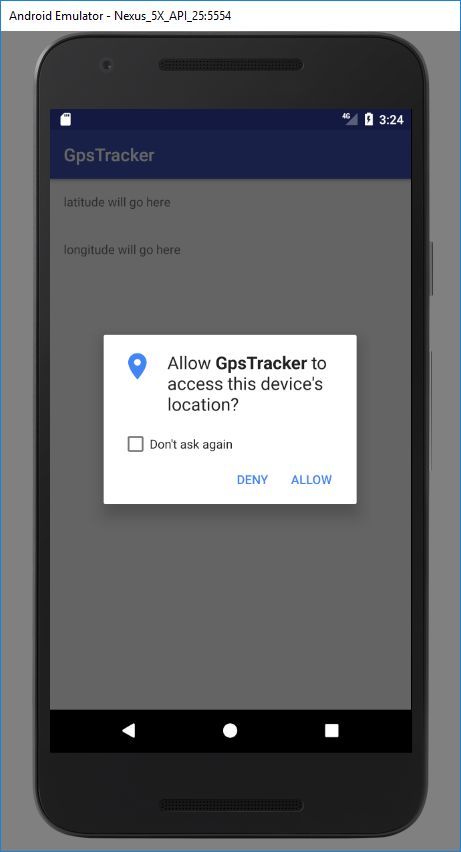
then next time can just launch without having to give permission:
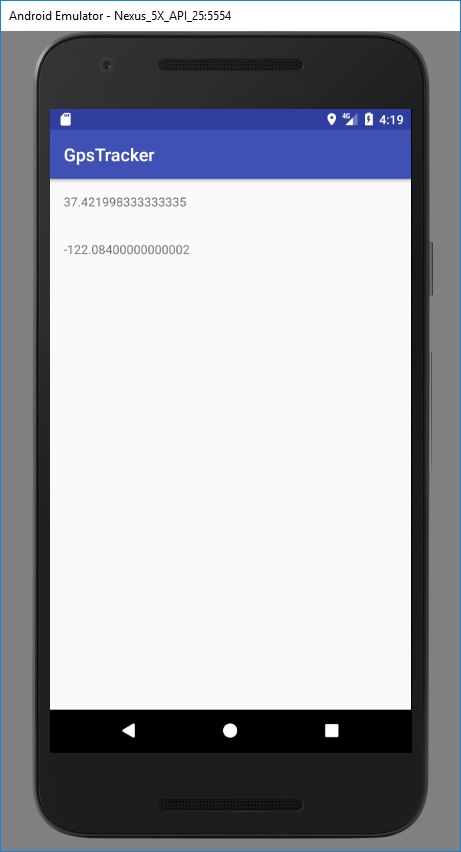
it took my Sony Xperia Z5 Compact at least 7 seconds before the GPS 'kicked in'. also on Android 4.1 and lower, the Developer options screen is available by default. On Android 4.2 and higher, you must enable this screen by going Settings > About phone > tap Build number 7 times.
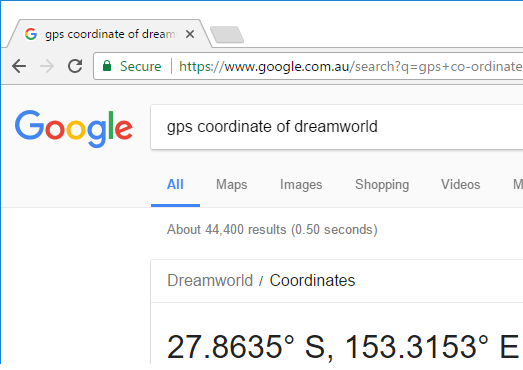