my calculator
Setup App
(remaining tutorials will assume the following steps have been completed):- click 'Start a new Android Studio project'
- set Application name: MyCalculator (for other tutorials use a different but suitable name, with no spaces)... and click 'Next'
- use default Phone and Tablet form factor from previous tutorial... and click 'Next'
- add an Empty Activity... and click 'Next'
- leave Activity Name as MainActivity and click 'Finish' (this is entry point of your app)
- hit Run 'app' play button at top of screen to check everything is working. run the app on any Available Virtual Device (hit OK)
- close emulator once app has launched successfully
Layout Editor
open app > res > layout > activity_main.xml
drag drop two Plain Text boxes onto user interface, set ID of each to txtNumOne and txtNumTwo, also set text to a suitable prompt:
drag drop a Button onto user interface, set ID to btnAdd. im using TextView object from "Hello World", either way set ID to txtAnswer, and once again add suitable prompts:
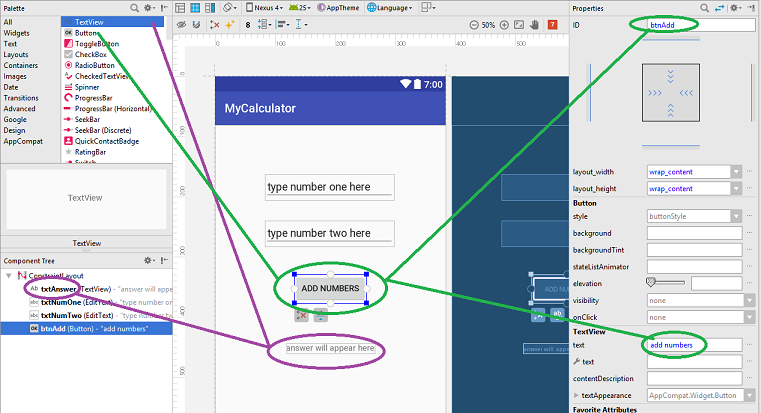
hit play - should get some bunching up:
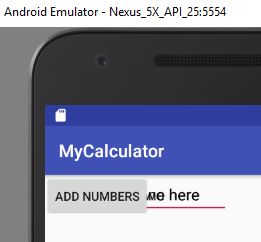
set constraints from top of layout - click, drag, release (object will snap to top), then pull object down. similiarly you can also set from left side of layout. you can also remove any constraint by right clicking on toggle and choosing Clear All Constraints:
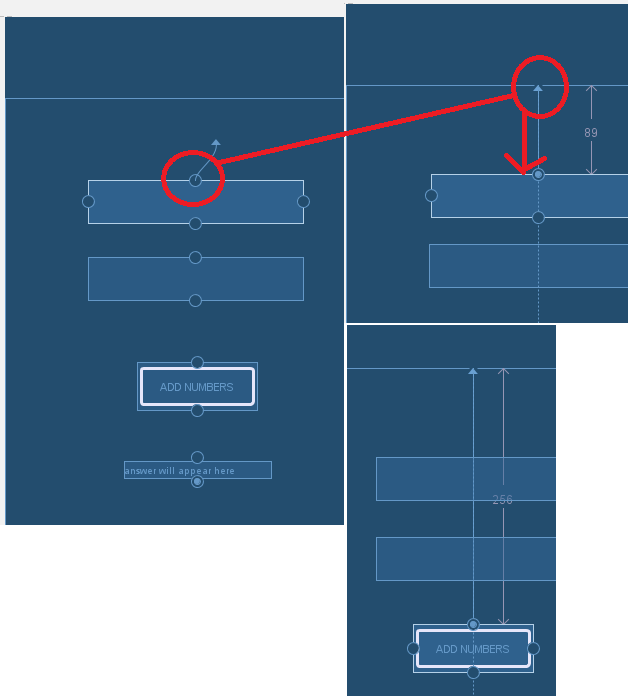
much better:
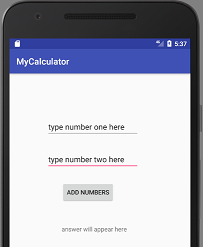
Code
goes in MainActivity.java:package com.example.my.mycalculator; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; //~~~~~~~~~~~~~~~~~~~~~~~~~~ //import necessary packages: import android.widget.EditText; import android.widget.TextView; import android.widget.Button; import android.view.View; //~~~~~~~~~~~~~~~~~~~~~~~~~~ public class MainActivity extends AppCompatActivity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); //~~~~~~~~~~~~~~~~~~~~~~~~~~~ //link btnAdd on user layout //to code using a 'listener': Button addNumbers = (Button) findViewById(R.id.btnAdd); addNumbers.setOnClickListener(allViewClicksGoHere); //~~~~~~~~~~~~~~~~~~~~~~~~~~~ } //~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ //creating a listener allViewClicksGoHere: private View.OnClickListener allViewClicksGoHere = new View.OnClickListener(){ public void onClick(View v) { //so we can be sure we've hit add: if(v.getId() == R.id.btnAdd) { //locate objects in view: EditText numberOne = (EditText) findViewById(R.id.htmlNumOne); EditText numberTwo = (EditText) findViewById(R.id.htmlNumTwo); TextView answer = (TextView) findViewById(R.id.htmlAnswer); //perform calculation: int num1 = Integer.parseInt(numberOne.getText().toString()); int num2 = Integer.parseInt(numberTwo.getText().toString()); int total = num1 + num2; answer.setText(String.valueOf(total)); } } }; //~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ }
Run
brilliant
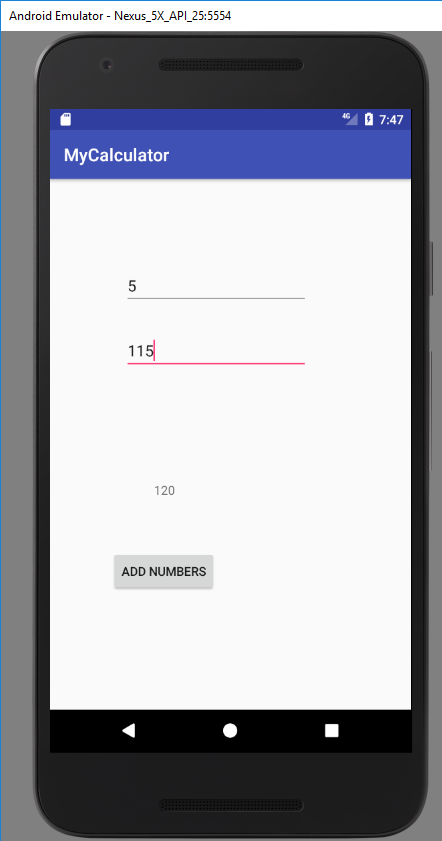