python 1
note to all programmers from other languages: indenting (using the TAB key) matters!
- install
- helloWorld
- addNumbers
- randoms
- headsTails
- post-testLoops
- pre-testLoops
- forLoops
- puttingItTogether
install
- download newest / latest python
- download pycharm community (free) edition EDIT: or just use IDLE (offline), repl.it or trinket (online), and skip these steps
- install both (python first), go with default settings when asked questions
- start pycharm, create new project
- choose location, find interpreter (where you installed python - should detect automatically)
- add a new python file, save it and give it a name
- try helloWorld script below, right-click run, or use run menu or shortcut key
- any issues use ^^find Edit Configurations... button
- ^^check if script path is correct
- ^^check if installed Python interpreter has been selected
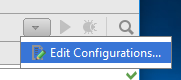


helloWorld
print("hello world")
addNumbers
num1 = int(input("enter first number: ")) num2 = int(input("enter second number: ")) answer = num1 + num2 print("the answer is: " + str(answer))
randoms
import random print(random.randint(1,6)) #between 1 & 6 inclusive print(random.uniform(0,1)) #between 0 & 1, floating
headsTails
console based:
import random if(random.randint(1,2)==1): print("heads") else: print("tails")... or using a function:
import random def flipCoinFunc(): if(random.randint(1,2)==1): print("heads") else: print("tails") flipCoinFunc()... or as a switch / case statement for java / C# programmers:
import random def flipCoinFunc(x): return { 1: "heads", 2: "tails" }[x] temp = random.randint(1,2) print(flipCoinFunc(temp))
graphically:
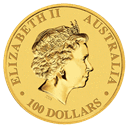
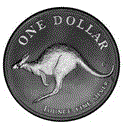
import random import tkinter #for python 3, python 2 would have a capital T instead myWindow = tkinter.Tk() imgHeads = tkinter.PhotoImage(file="heads.gif") imgTails = tkinter.PhotoImage(file="tails.gif") def flipCoinFunc(event): if(random.randint(1,2)==1): resultLabel.configure(image = imgHeads) else: resultLabel.configure(image = imgTails) btnFlip = tkinter.Button(myWindow, text="click to flip", fg="magenta") btnFlip.bind("<ButtonPress>",flipCoinFunc) btnFlip.pack(side="top") resultLabel = tkinter.Label(image = imgHeads) resultLabel.pack(side="top") myWindow.title("my coin toss app") myWindow.geometry("300x300") myWindow.configure(background='salmon') myWindow.mainloop()
post-test loops
repeats 1 or more times, not good practise!count = 10 while True: print(count) count=count-1 if(count < 1): break; print("blastoff!")
pre-test loops
repeats 0 or more times, use when num of iterations unknown:count = 10 while (count >= 1): print(count) count=count-1 print("blastoff!")
for loops
for more controlled looping, when num of iterations are known:for look in [1,3,5,7,9]: print(look) #range(start,stop,step): for test in range(10,0,-2): print(test) for x in range(1, 4): #1 to 3 only for y in range(1, 4): print(x, y) for x in "camelCase": print(x)
putting it together
simulating a "coin toss simulator", that tests how long it takes to toss consecutive heads / tails:import random countHeads = 0 countTails = 0 exitLoop = 7 #number of heads or tails in a row prevFlip = 0 flip = 0 numberOfFlips = 0 while (countHeads < exitLoop) and (countTails < exitLoop): prevFlip = flip flip = random.randint(1,2); numberOfFlips += 1 print("{face}".format(face="heads" if flip is 1 else "tails")) if(flip == prevFlip and flip == 1): countHeads+=1 if(flip == prevFlip and flip == 2): countTails+=1 if(flip != prevFlip): if(flip==1): countHeads = 1 countTails = 0 if(flip==2): countHeads = 0 countTails = 1 print("number of flips: ", numberOfFlips)